fmeobjects.FMEPalette
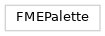
|
Adds keys and values to the palette. |
|
Returns whether the specified keys are contained in the palette. |
|
Returns whether the specified values are contained in the palette. |
|
Evaluates whether two palettes are identical. |
|
Returns the indices of the specified keys in the palette. |
Returns the keys of the palette. |
|
Returns a tile containing the maximum values of each component in the palette. |
|
Returns the maximum key of the palette. |
|
Returns the maximum value of the palette. |
|
Returns a tile containing the minimum values of each component in the palette. |
|
Returns the minimum key of the palette. |
|
Returns the minimum value of the palette. |
|
Returns the name of the palette. |
|
|
Returns the next available keys from the palette. |
Returns the number of entries in the palette. |
|
Returns the values of the palette. |
|
Returns resolved values from the keys parameter. |
|
Returns whether the palette has been modified from its baseline state. |
|
Returns whether the palette is selected for operations. |
|
Removes specified keys and corresponding values from the palette. |
|
|
Sets whether palette has been modified from baseline state. |
|
Selects/deselects palette for operations. |
|
Sets value associated with the key of the palette. |
- class FMEPalette
FME Palette Class
Create an instance of a
FMEPalette
object.init(name, key, value)
Creates a palette with the given properties.
The keys tile and values tile must have the same number of entries.
- Parameters:
- Return type:
- Returns:
An instance of a Palette object.
init(palette)
Create a copy of the passed in palette object.
- Parameters:
palette (FMEPalette) – The palette object to create a copy of.
- Return type:
- Returns:
An instance of a
FMEPalette
object.
- __init__(*args, **kwargs)
- addKeysAndValues(keys, values)
Adds keys and values to the palette. This method can add and/or set multiple values at once.
The input keys tile must be the same interpretation as the palette keys. The input values tile must be the same interpretation as the palette values.
The size of keys must be the same as the size of the values.
- Parameters:
- Return type:
None
- Raises:
An exception is raised:
if an error occurred.
if the number of new keys exceed the maximum number of keys this palette can handle.
- containsKeys(keys)
Returns whether the specified keys are contained in the palette. The input tile may contain multiple keys.
- containsValues(values)
Returns whether the specified values are contained in the palette. The input tile may contain multiple values.
- equals(palette)
Evaluates whether two palettes are identical.
- Parameters:
palette (FMEPalette) – The palette object to compare to.
- Return type:
- Returns:
True
if the two palette are the same.
- getKeyIndices(keys)
Returns the indices of the specified keys in the palette.
An index represents the position of a key in the overall list of keys. For example, if a palette contains the following keys: 0 2 5 8, then the corresponding indices are: 0 1 2 3.
- Parameters:
keys (FMETile) – The keys tiles to look for. The keys tiles must be the same interpretation as the palette keys.
- Return type:
- Returns:
Indices of the specified keys in the palette.
- Raises:
An exception is raised:
if an error occurred.
if the palette does not contain one of the specified keys.
- getKeyTile()
Returns the keys of the palette.
- Return type:
- Returns:
Keys of the palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getMaxComponentValues()
Returns a tile containing the maximum values of each component in the palette.
This tile might not be part of the palette itself and might not have a corresponding key tile.
- Return type:
- Returns:
A tile containing the maximum value of each component in this palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getMaxKey()
Returns the maximum key of the palette.
- Return type:
- Returns:
The maximum key of the palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getMaxValue()
Returns the maximum value of the palette.
- Return type:
- Returns:
The maximum value of the palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getMinComponentValues()
Returns a tile containing the minimum values of each component in the palette.
This tile might not be part of the palette itself and might not have a corresponding key tile.
- Return type:
- Returns:
A tile containing the minimum value of each component in this palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getMinKey()
Returns the minimum key of the palette.
- Return type:
- Returns:
The minimum key of the palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getMinValue()
Returns the minimum value of the palette.
- Return type:
- Returns:
The minimum value of the palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getNextAvailableKeys(numKeys)
Returns the next available keys from the palette.
This method can get multiple available keys at once.
This routine can be used before the
addKeysAndValues
routine to make sure that there are enough new keys.- Parameters:
numKeys (int) – Number of keys to look up.
- Return type:
- Returns:
The next available keys from the palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getNumEntries()
Returns the number of entries in the palette.
- Return type:
- Returns:
The number of entries in the palette.
- getValueTile()
Returns the values of the palette.
- Return type:
- Returns:
Values of the palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- getValuesFromKeys(keys)
Returns resolved values from the keys parameter.
This method allow multiple key-to-value lookups.
The input keys tile must be the same interpretation as the palette keys.
- Parameters:
keys (FMETile) – Input key tile. It must be the same interpretation as the palette keys.
- Return type:
- Returns:
Values associated with keys. If specified key is not found in the palette, the value will be set to 0.
- Raises:
FMEException – An exception is raised if an error occurred.
- hasBeenModified()
Returns whether the palette has been modified from its baseline state.
- isSelected()
Returns whether the palette is selected for operations.
- removeKeysAndValues(keys)
Removes specified keys and corresponding values from the palette. This method can remove multiple keys and values at once.
The input keys tile must be the same interpretation as the palette keys.
Note that palette cannot be empty, meaning that there must be at least one entry in the palette. Therefore, attempting to remove everything from the palette will give an error and raise an exception.
- Parameters:
keys (FMETile) – Key to remove.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setModified(modified)
Sets whether palette has been modified from baseline state.
Note that
hasBeenModified
is initially set toFalse
. Then, any calls that alter the palette will cause the modified state to becomeTrue
(this does not need to be explicitly set; it will occur automatically).
- setSelected(selected)
Selects/deselects palette for operations.
- setValuesFromKeys(keys, values)
Sets value associated with the key of the palette. This method can set multiple values at once.
The input keys tile must be the same interpretation as the palette keys. The input values tile must be the same interpretation as the palette values.
The size of the keys must be the same as the size of the values.
- Parameters:
- Return type:
None
- Raises:
An exception is raised:
if an error occurred.
if some of the keys do not exist.