fmeobjects.FMERasterProperties
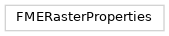
|
This method evaluates whether two properties have the identical value. |
This method returns origin, spacing rotation as an affine transformation. |
|
Get the horizontal position of the cell origin within each cell. |
|
Get the vertical position of the cell origin within each cell. |
|
Get OGC definition of the GCP coordinate system. |
|
Get the number of GCPs stored on the raster geometry. |
|
Get a list of dictionaries where each dictionary contains 'row', 'col', 'x', 'y', and 'z' (optional). |
|
Get the x ground coordinate value of the lower-left corner of the unrotated raster, after rotation is applied. |
|
Get the y ground coordinate value of the lower-left y corner of the unrotated raster, after rotation is applied. |
|
Get the x ground coordinate value of the lower-right corner of the unrotated raster, after rotation is applied. |
|
Get the y ground coordinate value of the lower-right corner of the unrotated raster, after rotation is applied. |
|
Get the x ground coordinate value of the right-most side of the right-most cell of the raster. |
|
Get the y ground coordinate value of the top-most side of the top-most cell of the raster. |
|
Get the x ground coordinate value of the left-most side of the left-most cell of the raster. |
|
Get the y ground coordinate value of the bottom-most side of the bottom-most cell of the raster. |
|
Get the number of columns in the raster. |
|
Get the number of rows in the raster. |
|
Get the x coordinate of the origin or the raster. |
|
Get the y coordinate of the origin or the raster. |
|
Get the rotation angle of the raster in radians from x axis. |
|
Get the rotation angle of the raster in radians from y axis. |
|
Get fixed distance in the x dimension between each pixel in the raster. |
|
Get fixed distance in the y dimension between each pixel in the raster. |
|
Get the ground x coordinate value of the upper-left corner of the unrotated raster, after rotation is applied. |
|
Get the ground y coordinate value of the upper-left corner of the unrotated raster, after rotation is applied. |
|
Get the x coordinate value of the upper-right corner of the unrotated raster, after rotation is applied. |
|
Get the y coordinate value of the upper-right corner of the unrotated raster, after rotation is applied. |
|
This method evaluates whether the raster properties have been modified from their baseline state. |
|
This method sets origin, spacing, and rotation as an affine transformation. |
|
|
This method sets horizontal position of the cell origin within each cell. |
|
This method sets vertical position of the cell origin within each cell. |
|
This method sets GCP coordinate system. |
|
This method sets a list of GCPs on the properties. |
|
This method sets whether raster properties have been modified from baseline state. |
|
This method sets number of columns of the raster. |
|
This method sets number of rows of the raster. |
|
This method sets x value of the upper left corner of the raster in ground coordinates. |
|
This method sets y value of the upper left corner of the raster in ground coordinates. |
|
This method sets the rotation angle of the raster in radians as measured in a CCW direction. |
setRotationY(yRotation) |
|
|
setRotationX(xRotation) |
|
This method sets the x spacing (cell size) of the raster. |
|
This method sets the y spacing (cell size) of the raster |
- class FMERasterProperties
__getObject__()
FME Raster Properties Class
Create an instance of a
FMERasterProperties
object.init(numRows, numCols, xSpacing, ySpacing, xCellOrigin, yCellOrigin, xOrigin, yOrigin, xRotation, yRotation)
Creates a raster property with the given properties.
- Parameters:
numRows (int) – Number of Rows. Must be positive integer.
numCols (int) – Number of Columns. Must be positive integer.
xSpacing (float) – Space between columns. Must be positive float.
ySpacing (float) – Space between rows. Must be positive float.
xCellOrigin (float) – Cell origin within a cell: X coordinate. Must be between 0 to 1, inclusive.
yCellOrigin (float) – Cell origin within a cell: Y coodinate. Must be between 0 to 1, inclusive.
xOrigin (float) – x value of the upper left corner of the raster.
yOrigin (float) – y value of the upper left corner of the raster.
xRotation (float) – Rotation angle of the raster in radians as measured in a CCW direction from the positive x axis.
yRotation (float) – Rotation angle of the raster in radians as measured in a CCW direction from the negative y axis.
- Return type:
- Returns:
An instance of a
RasterProperties
object.
init(numRows, numCols, xCellOrigin, yCellOrigin, transform)
Creates a raster properties object from an affine transformation.
- Parameters:
numRows (int) – Number of Rows. Must be positive integer.
numCols (int) – Number of Columns. Must be positive integer.
xCellOrigin (float) – Cell origin within a cell: X coordinate. Must be between 0 to 1, inclusive.
yCellOrigin (float) – Cell origin within a cell: Y coodinate. Must be between 0 to 1, inclusive.
transform (list) – List containing 6 Coefficients. A B C D E F in the affine transformation x’ = Ax + By + C, y’ = Dx + Ey + F.
- Return type:
- Returns:
An instance of a
RasterProperties
object.
init(rasterProperties)
Create a copy of the passed in raster properties object.
- Parameters:
rasterProperties (FMERasterProperties) – The rasterProperties object to create a copy of.
- Return type:
- Returns:
An instance of a
RasterProperties
object.
- __init__(*args, **kwargs)
- equals(fmerp)
This method evaluates whether two properties have the identical value.
- Parameters:
fmerp (FMERasterProperties) – The raster property to compare to.
- Return type:
- Returns:
True
if the two properties had the same value.
- getAsAffineTransform()
This method returns origin, spacing rotation as an affine transformation.
- getCellOriginX()
Get the horizontal position of the cell origin within each cell.
- Return type:
- Returns:
The horizontal position of the cell origin.
- getCellOriginY()
Get the vertical position of the cell origin within each cell.
- Return type:
- Returns:
The vertical position of the cell origin.
- getGCPCoordSys()
Get OGC definition of the GCP coordinate system.
- Return type:
- Returns:
OGC definition of the GCP coordinate system.
- getGCPCount()
Get the number of GCPs stored on the raster geometry.
- Return type:
- Returns:
The number of GCPs stored on the raster geometry.
- getGCPs()
Get a list of dictionaries where each dictionary contains ‘row’, ‘col’, ‘x’, ‘y’, and ‘z’ (optional).
When ‘z’ is not set, value of ‘z’ will be set to 0.
- getLowerLeftCornerX()
Get the x ground coordinate value of the lower-left corner of the unrotated raster, after rotation is applied.
- getLowerLeftCornerY()
Get the y ground coordinate value of the lower-left y corner of the unrotated raster, after rotation is applied.
- getLowerRightCornerX()
Get the x ground coordinate value of the lower-right corner of the unrotated raster, after rotation is applied.
- getLowerRightCornerY()
Get the y ground coordinate value of the lower-right corner of the unrotated raster, after rotation is applied.
- getMaxX()
Get the x ground coordinate value of the right-most side of the right-most cell of the raster.
- Return type:
- Returns:
Ground coordinate value of the right-most side of the right-most cell of the raster.
- getMaxY()
Get the y ground coordinate value of the top-most side of the top-most cell of the raster.
- Return type:
- Returns:
Ground coordinate value of the top-most side of the right-most cell of the raster.
- getMinX()
Get the x ground coordinate value of the left-most side of the left-most cell of the raster.
- Return type:
- Returns:
Ground coordinate value of the left-most side of the left-most cell of the raster.
- getMinY()
Get the y ground coordinate value of the bottom-most side of the bottom-most cell of the raster.
- Return type:
- Returns:
Ground coordinate value of the bottom-most side of the bottom-most cell of the raster.
- getNumCols()
Get the number of columns in the raster.
- Return type:
- Returns:
The number of columns.
- getOriginX()
Get the x coordinate of the origin or the raster.
- Return type:
- Returns:
X coordinate of the raster. It’s the ground coordinate value of the top-left cell in the top-left corner of the raster.
- getOriginY()
Get the y coordinate of the origin or the raster.
- Return type:
- Returns:
Y coordinate of the raster. It’s the ground coordinate value of the top-left cell in the top-left corner of the raster.
- getRotationX()
Get the rotation angle of the raster in radians from x axis.
- Return type:
- Returns:
Rotation angle of the raster in radians. Measured in a CCW direction from the positive x axis.
- getRotationY()
Get the rotation angle of the raster in radians from y axis.
- Return type:
- Returns:
Rotation angle of the raster in radians. Measured in a CCW direction from the negative y axis.
- getSpacingX()
Get fixed distance in the x dimension between each pixel in the raster.
- Return type:
- Returns:
x spacing (cell size) of the raster.
- getSpacingY()
Get fixed distance in the y dimension between each pixel in the raster.
- Return type:
- Returns:
y spacing (cell size) of the raster.
- getUpperLeftCornerX()
Get the ground x coordinate value of the upper-left corner of the unrotated raster, after rotation is applied.
- getUpperLeftCornerY()
Get the ground y coordinate value of the upper-left corner of the unrotated raster, after rotation is applied.
- getUpperRightCornerX()
Get the x coordinate value of the upper-right corner of the unrotated raster, after rotation is applied.
- getUpperRightCornerY()
Get the y coordinate value of the upper-right corner of the unrotated raster, after rotation is applied.
- hasBeenModified()
This method evaluates whether the raster properties have been modified from their baseline state.
- setAsAffineTransform(affineTransform)
This method sets origin, spacing, and rotation as an affine transformation.
- setCellOriginX(xCellOrigin)
This method sets horizontal position of the cell origin within each cell.
Once cell origin is specified, it applies to all cells.
- Parameters:
xCellOrigin (float) – horizontal position of the cell origin within each cell. Cell origin is a normalized value so the value should be in the range of 0.0 to 1.0 inclusive. For example, cell with origin set to (0.5, 0.5) will have its origin at the center.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setCellOriginY(yCellOrigin)
This method sets vertical position of the cell origin within each cell.
Once cell origin is specified, it applies to all cells.
- Parameters:
yCellOrigin (float) – vertical position of the cell origin within each cell. Cell origin is a normalized value so the value should be in the range of 0.0 to 1.0 inclusive. For example, cell with origin set to (0.5, 0.5) will have its origin at the center.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setGCPCoordSys(gcpCoordSys)
This method sets GCP coordinate system.
- Parameters:
gcpCoordSys (str) – GCP coordinate system.
- Return type:
None
- setGCPs(dataList, coordSys)
This method sets a list of GCPs on the properties.
- Parameters:
dataList (list[dict]) – A list of dictionaries where each dictionary contains row, col, x, y, and z (optional). For example, to set all of the keys, a list should look like as follows: [{‘row’:1, ‘col’:2, ‘x’:3, ‘y’:4, ‘z’:5}]. To set row, col, x, and y only, do not specify ‘z’ in the list: [{‘row’:1, ‘col’:2, ‘x’:3, ‘y’:4}]. If z is not specified, it will be set to 0.
coordSys (str) – GCP coordinate system.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setModified(modified)
This method sets whether raster properties have been modified from baseline state.
Note that
hasBeenModified()
is initially set toFalse
.
- setNumCols(numCols)
This method sets number of columns of the raster.
- Parameters:
numCols (int) – Number of columns of the raster. Number must be a positive integer.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setNumRows(numRows)
This method sets number of rows of the raster.
- Parameters:
numRows (int) – Number of rows of the raster. Number must be a positive integer.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setOriginX(xOrigin)
This method sets x value of the upper left corner of the raster in ground coordinates.
- Parameters:
xOrigin (float) – x value of the upper left corner of the raster.
- Return type:
None
- setOriginY(yOrigin)
This method sets y value of the upper left corner of the raster in ground coordinates.
- Parameters:
yOrigin (float) – y value of the upper left corner of the raster.
- Return type:
None
- setRotation(rotation)
This method sets the rotation angle of the raster in radians as measured in a CCW direction.
- Parameters:
rotation (float) – Rotation angle of the raster in radians as measured in a CCW direction.
- Return type:
None
- setRotationX()
setRotationY(yRotation)
setRotationX(xRotation)
This method sets the rotation angle of the raster in radians.
- Parameters:
xRotation (float) – Rotation angle of the raster in radians as measured in a CCW direction from the positive x axis.
- Return type:
None
- setRotationY(yRotation)
setRotationX(xRotation)
This method sets the rotation angle of the raster in radians.
- Parameters:
yRotation (float) – Rotation angle of the raster in radians as measured in a CCW direction from the negative y axis.
- Return type:
None
- setSpacingX(xSpacing)
This method sets the x spacing (cell size) of the raster.
- Parameters:
xSpacing (float) – Fixed distance in the x dimension between each pixel in the raster. Number must be positive value.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setSpacingY(ySpacing)
This method sets the y spacing (cell size) of the raster
- Parameters:
ySpacing (float) – Fixed distance in the y dimension between each pixel in the raster. Number must be positive value.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.