fmeobjects.FMETexture
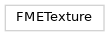
This routine makes a duplicate of itself. |
|
This routine retrieves the border color of this Texture. |
|
This routine retrieves the center associated with the texture. |
|
This routine retrieves the offset associated with the texture. |
|
This routine retrieves the rotation angle associated with the texture. |
|
This routine retrieves the scaling factors associated with the texture. |
|
This routine retrieves the shearing factors associated with the texture. |
|
This routine retrieves the wrapping style associated with the texture. |
|
This routine retrieves the texture transformation matrix associated with the texture, it is a combination of the rotation, scaling, shear and translation factors, applied in that order. |
|
This routine retrieves the texture transformation matrix associated with the texture in the reverse order and inverse of each operation, that consists of offset, negative shear, 1 / scale, and negative rotation in that order. |
|
This routine returns |
|
|
This routine sets the border color of this Texture. |
|
This routine sets the center associated with the texture. |
|
This routine sets the offset associated with the texture. |
|
This routine sets the rotation angle associated with the texture. |
|
This routine sets the scaling factors associated with the texture. |
|
This routine sets the shearing factors associated with the texture. |
|
This routine sets the wrapping style associated with the texture. |
This routine sets the texture transformation matrix associated with the texture. |
- class FMETexture
FME Texture Class
init()
Create an instance of a blank texture object.
- __init__(*args, **kwargs)
- clone()
This routine makes a duplicate of itself.
- Return type:
- Returns:
Returns a duplicate of the texture.
- getBorderColor()
This routine retrieves the border color of this Texture. The color is a vector in r g b color space with values between 0.0 and 1.0. This will return
None
if it did not have a border color set.
- getCenter()
This routine retrieves the center associated with the texture. This will return
None
if the center is the default center of (0,0). The center is only used for shearing and rotation.
- getOffset()
This routine retrieves the offset associated with the texture. This will return
None
if the offset is the default offset of (0,0).
- getRotation()
This routine retrieves the rotation angle associated with the texture. The angle is stored in degrees CCW from the x-axis, and is by default 0. Rotation is centered according to the Center variable. This return
None
if the scaling is the default angle of 0.- Return type:
- Returns:
The angle of rotation.
- getScaling()
This routine retrieves the scaling factors associated with the texture. This will return
None
if the scaling is the default scaling of (1,1).
- getShear()
This routine retrieves the shearing factors associated with the texture. Shearing is centered according to the Center variable. This will return
None
if the shearing is the default shearing of (0,0).
- getTextureWrap()
This routine retrieves the wrapping style associated with the texture. The style is stored as a Texture Wrap value, and is by default
FME_TEXTURE_REPEAT_BOTH
, which results in the texture being tiled in both the u and v direction.- Return type:
- Returns:
The wrapping style associated with the texture. One of :
FME_TEXTURE_BORDER_FILL
,FME_TEXTURE_REPEAT_BOTH
,FME_TEXTURE_CLAMP_BOTH
, :py:obj`FME_TEXTURE_CLAMP_U_REPEAT_V`,FME_TEXTURE_REPEAT_U_CLAMP_V
,FME_TEXTURE_MIRROR
,FME_TEXTURE_NONE
.
- getTransformationMatrix()
This routine retrieves the texture transformation matrix associated with the texture, it is a combination of the rotation, scaling, shear and translation factors, applied in that order.
- getTransformationMatrixReverse()
This routine retrieves the texture transformation matrix associated with the texture in the reverse order and inverse of each operation, that consists of offset, negative shear, 1 / scale, and negative rotation in that order. This matrix can be used to transform the texture coordinates that are associated with this texture.
- hasNonIdentityTransform()
This routine returns
True
if any non-default texture transform factors are set. The default texture transform matrix is identity and this returnsFalse
.
- setBorderColor(r, g, b)
This routine sets the border color of this Texture. The color values should be in r g b color space with values between 0.0 and 1.0. The border color is used only if the Texture Wrap value is set to
FME_TEXTURE_BORDER_FILL
. Setting the border color will also set the Texture Wrap value toFME_TEXTURE_BORDER_FILL
. Setting the any color value to a negative value will remove the color from this Texture and the Texture Wrap value will be reset toFME_TEXTURE_REPEAT_BOTH
. If the underlying raster is single channel, the gray-scale equivalent border color will be calculated.
- setCenter(u, v)
This routine sets the center associated with the texture. The default center for a texture is (0,0). The center is only used for shearing and rotation.
- setOffset(u, v)
This routine sets the offset associated with the texture. The default offset for a texture is (0,0).
- setRotation(angle)
This routine sets the rotation angle associated with the texture. Rotation is centered according to the Center variable. The angle is stored in degrees CCW from the x-axis, and is by default 0.
- Parameters:
angle (float) – The angle of the rotation.
- Return type:
None
- setScaling(u, v)
This routine sets the scaling factors associated with the texture. The default scaling for a texture is (1,1).
- setShear(u, v)
This routine sets the shearing factors associated with the texture. Shearing is centered according to the Center variable. The default shearing for a texture is (0,0).
- setTextureWrap(wrapStyle)
This routine sets the wrapping style associated with the texture. The style is stored as a Texture Wrap value. If the style is set to
FME_TEXTURE_BORDER_FILL
, the default border color of black (0,0,0), will be set. If the style is set to something other thanFME_TEXTURE_BORDER_FILL
, the border color will be removed from the texture.- Parameters:
wrapStyle (int) – The wrapping style associated with the texture. One of :
FME_TEXTURE_BORDER_FILL
,FME_TEXTURE_REPEAT_BOTH
,FME_TEXTURE_CLAMP_BOTH
,FME_TEXTURE_CLAMP_U_REPEAT_V
,FME_TEXTURE_REPEAT_U_CLAMP_V
,FME_TEXTURE_MIRROR
,FME_TEXTURE_NONE
.- Return type:
None
- setTransformationMatrix(matrix)
This routine sets the texture transformation matrix associated with the texture. The shear, offset, rotation and will be calculated from the matrix so that when rotation, shear, scale and translation are applied in order, it is the equivalent of the input matrix. If the non-translation component of the matrix has a zero determinant or m[0][0] = 0, the matrix will not be used and existing parameters will remain unchanged.