fmeobjects.FMEAppearance
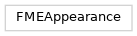
This routine retrieves the alpha (1-transparency) of this Appearance. |
|
This routine retrieves the ambient color of this Appearance as r g b values between 0.0 and 1.0. |
|
This routine retrieves the diffuse color of this Appearance as r g b values between 0.0 and 1.0. |
|
This routine retrieves the emissive color the color of this Appearance as r g b values between 0.0 and 1.0. |
|
This routine retrieves the specular color specular of this Appearance as r g b values between 0.0 and 1.0 This will return |
|
getMapperReference(mapperType), |
|
This routine retrieves the 'name' of this Appearance as a |
|
This routine retrieves the Shininess of this Appearance. |
|
This routine retrieves the main texture reference of this Appearance. |
|
removeMapperReference(mapperType), |
|
This routine removes the main texture reference from this Appearance. |
|
setAlpha(alpha), |
|
|
This routine sets the Ambient color of this Appearance. |
|
This routine sets the Diffuse color of this Appearance. |
|
This routine sets the Emissive color of this Appearance. |
|
This routine sets the Specular color of this Appearance. |
|
This routine sets a Mapper that modifies this Appearance. |
setName(name), |
|
setShininess(shininess), |
|
setTextureReference(textureReference), |
- class FMEAppearance
FME Appearance Class
init()
Create an instance of a blank appearance object.
- __init__(*args, **kwargs)
- getAlpha()
This routine retrieves the alpha (1-transparency) of this Appearance. This will return
None
if it did not have a alpha associated with it.- Return type:
float or None
- Returns:
The alpha of this appearance.
- getColorAmbient()
This routine retrieves the ambient color of this Appearance as r g b values between 0.0 and 1.0. This will return
None
if it did not have an ambient color associated with it.
- getColorDiffuse()
This routine retrieves the diffuse color of this Appearance as r g b values between 0.0 and 1.0. This will return
None
if it did not have a diffuse color associated with it.
- getColorEmissive()
This routine retrieves the emissive color the color of this Appearance as r g b values between 0.0 and 1.0. This will return
None
if it did not have an emissive color associated with it.
- getColorSpecular()
This routine retrieves the specular color specular of this Appearance as r g b values between 0.0 and 1.0 This will return
None
if it did not have a specular color associated with it.
- getMapperReference()
getMapperReference(mapperType),
This routine retrieves the texture reference of ‘mapperType’ of this Appearance. This will return
None
if it did not have a mapper of mapper type associated with it.- Parameters:
mapperType (int) – The mapper type to retrieve the reference for. One of:
FME_MAPPER_ALPHA_MAP
,FME_MAPPER_ALPHA_MASK
,FME_MAPPER_AMBIENT_MAP
,FME_MAPPER_AMBIENT_MASK
,FME_MAPPER_DIFFUSE_MAP
,FME_MAPPER_DIFFUSE_MASK
,FME_MAPPER_EMISSIVE_MAP
,FME_MAPPER_EMISSIVE_MASK
,FME_MAPPER_SHININESS_MAP
,FME_MAPPER_SHININESS_MASK
,FME_MAPPER_SPECULAR_MAP
,FME_MAPPER_SPECULAR_MASK
,FME_MAPPER_BUMP_MAP
, ORFME_MAPPER_BUMP_MASK
.- Return type:
int or None
- Returns:
The texture reference of ‘mapperType’ of this Appearance.
- getName()
This routine retrieves the ‘name’ of this Appearance as a
str
. This will returnNone
if it did not have a name associated with it.- Return type:
str or None
- Returns:
The appearance’s name.
- getShininess()
This routine retrieves the Shininess of this Appearance. This will return
None
if it did not have a shininess associated with it.
- getTextureReference()
This routine retrieves the main texture reference of this Appearance. This will return
None
if it did not have a texture reference associated with it.
- removeMapperReference()
removeMapperReference(mapperType),
This routine removes the specified mapper reference from this Appearance.
- Parameters:
mapperType (int) – The mapper reference to remove.
- Return type:
None
- removeTextureReference()
This routine removes the main texture reference from this Appearance.
- Return type:
None
- setAlpha()
setAlpha(alpha),
This routine sets the alpha of this Appearance. The alpha should be a floating point value in the range of 0.0 to 1.0, where 0.0 represents a fully transparent color, and 1.0 represents a fully opaque color. Setting the value to negative will remove this property from this appearance.
- Parameters:
alpha (float) – The alpha value to set on the appearance.
- Return type:
None
- setColorAmbient(r, g, b), b)
This routine sets the Ambient color of this Appearance. The color values should be in r g b color space with values between 0.0 and 1.0. Setting any color value to a negative value will remove the color from this Appearance.
- setColorDiffuse(r, g, b)
This routine sets the Diffuse color of this Appearance. The color values should be in r g b color space with values between 0.0 and 1.0. Setting any color value to a negative value will remove the color from this Appearance.
- setColorEmissive(r, g, b)
This routine sets the Emissive color of this Appearance. The color values should be in r g b color space with values between 0.0 and 1.0. Setting any color value to a negative value will remove the color from this Appearance.
- setColorSpecular(r, g, b)
This routine sets the Specular color of this Appearance. The color values should be in r g b color space with values between 0.0 and 1.0. Setting any color value to a negative value will remove the color from this Appearance.
- setMapperReference(mapperType, textureReference)
This routine sets a Mapper that modifies this Appearance. The Mapper is a texture object that modifies some property of the Appearance. One of:
FME_MAPPER_ALPHA_MAP
,FME_MAPPER_ALPHA_MASK
,FME_MAPPER_AMBIENT_MAP
,FME_MAPPER_AMBIENT_MASK
,FME_MAPPER_DIFFUSE_MAP
,FME_MAPPER_DIFFUSE_MASK
,FME_MAPPER_EMISSIVE_MAP
,FME_MAPPER_EMISSIVE_MASK
,FME_MAPPER_SHININESS_MAP
,FME_MAPPER_SHININESS_MASK
,FME_MAPPER_SPECULAR_MAP
,FME_MAPPER_SPECULAR_MASK
,FME_MAPPER_BUMP_MAP
, ORFME_MAPPER_BUMP_MASK
. The ‘textureReference’ should refer to a Texture found in the commonFMELibrary
.True
will be returned if the reference supplied refers to a valid Texture in the library,False
indicates a hanging reference.
- setName()
setName(name),
This routine sets the ‘name’ of this Appearance with a
str
. (It need not be a unique name among other, possibly different Appearances.) Setting the ‘name’ to be (blank) will remove the name from this Appearance.- Parameters:
name (str) – The name to set on this appearance.
- Return type:
None
- setShininess()
setShininess(shininess),
This routine sets the Shininess of this Appearance. The Shininess should be a floating point value in the range of 0.0 to 1.0. Setting the value to negative will remove this property from this appearance.
- Parameters:
shininess (float) – The shininess to set on the appearance.
- Return type:
None
- setTextureReference()
setTextureReference(textureReference),
This routine sets the main texture reference of this Appearance. The texture reference should be valid reference to a Texture found in the common
FMELibrary
. True will be returned if the reference supplied refers to a valid Texture in the library, false indicates a hanging reference.