fmeobjects.FMEGeometryTools
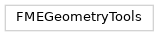
Appends the source curve to the destination curve. |
|
|
Apply the transformation matrix of the texture to the texture coordinates if exists and that it does not have any shear if 'applyOnlyIfShearExists' is set to |
This method returns |
|
This routine is to see if the current arc is closed. |
|
closeCurve(curve), |
|
|
This function closes the curve in 3D if it is not already closed. |
Returns a line that is created from the given curve. |
|
createXMLFromGeometry(geom), |
|
Extends the source curve to the destination curve. |
|
|
This method will extend the given curve to the given point. |
This routine consumes the text passed in and returns the |
|
|
This routine forces the geometry to 2D. |
|
Return the number of parts in the geometry. |
|
This routine joins two geometries together. |
makeDonuts(multiArea), |
|
|
This method performs a 3D matrix transformation on the geometry passed in. |
|
Offsets the geometry by the coords specified by 'point'. |
|
See the documentation for |
|
See the documentation for |
|
This routine offers a number of options to refine geometries. |
|
The angle is CCW up from the horizontal and is measured in degrees. |
|
The 'zscale' is ignored if geometry is 2D. |
|
This method is deprecated as of FME 2022.1. |
|
This method is deprecated as of FME 2022.1. |
|
This method is deprecated as of FME 2022.1. |
|
This method is deprecated as of FME 2022.1. |
splitDoubleSidedSurface(doubleSidedSurface), |
|
triangulateToSurface(geometry), |
- class FMEGeometryTools
FME Geometry Tools Class
init()
This class provides the ability to modify FME Geometry.
- __init__(*args, **kwargs)
- appendCurve(destinationCurve, sourceCurve)
Appends the source curve to the destination curve. This will not merge curves but simply append them into an
IFMEPath
if necessary. If the source curve isNone
, nothing will be done and the destination curve will be returned. If the destination curve isNone
, it will be set to the source curve. If the source and destination curves do not have touching end points, a line will be inserted to connect them.
- applyTransformationToTextureCoordinates(surface, applyOnlyIfShearExists)
Apply the transformation matrix of the texture to the texture coordinates if exists and that it does not have any shear if ‘applyOnlyIfShearExists’ is set to
true
. Note: This method should only be called once for one surface, or else the transformation matrix is applied as many times as the method is called.- Parameters:
surface (FMESurface) – The surface to apply the transformation to.
applyOnlyIfShearExists (bool) – If true only applies the transformation when a shear exists.
- Return type:
None
- calculateVertexNormals(repairOnlyMissing, repairType, geom)
This method returns
None
if vertex normals are repaired on the geometry or if there is nothing to repair. If an error occurs during the repair aFMEException
is thrown and the geom object is no longer valid for access. Currently, the only valid value for repairOnlyMissing is true, and the only valid value for repairType isBY_FACE
.- Parameters:
repairOnlyMissing (bool) – Boolean indicating whether or not to repair only the missing vertex normals, currently, the only true is valid.
repairType (int) – Repair type, the only valid value is
BY_FACE
.geom (FMEGeometry) – The geometry to calculate the vertex normals for.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- closeArcAsEllipse(arc)
This routine is to see if the current arc is closed. A closed arc is defined as one where the start and end points are equal. If
None
is passed in, nothing will be done. The returned geometry may or may not be the original object that was passed in. If a different object is returned, the original object will be destroyed.- Parameters:
arc (FMEArc) – The arc to close as an ellipse.
- Return type:
FMEEllipse or None
- Returns:
The Arc closed as an ellipse or
None
- closeCurve()
closeCurve(curve),
This checks to see if the current curve is closed. A closed curve is defined as one where the start and end points are equal. If necessary this method will add a straight segment from the end point to the start point to force the curve closed. If
None
is passed in, nothing will be done andNone
will be returned. The returned geometry may or may not be the original object that was passed in. If a different object is returned, the original object will be destroyed.- Parameters:
curve (FMECurve or None) – The curve to close.
- Return type:
FMECurve or None
- Returns:
The closed curve. Note: This method returns a terminal geometry type of the
FMECurve
; i.e. one of the leaf classes in theFMECurve
inheritance graph. For example, aFMEArc
.- Raises:
FMEException – An exception is raised if an error occurred.
- closeCurve3D(curve, mode)
This function closes the curve in 3D if it is not already closed. If the curve is not 3D, it forces the curve to be 3D with default z value of 0.0. A closed curve is defined as one where the start and end points are equal in all X, Y and Z coordinates. The returned geometry may or may not be the original object that was passed in. If
None
is passed in, nothing will be done andNone
will be returned. If a different object is returned, the original object will be destroyed. There are 3 modes that the function uses in deciding how to close the curve, namelyFME_CLOSE_3D_EXTEND_MODE
,FME_CLOSE_3D_AVERAGE_MODE
andFME_CLOSE_3D_EXTEND_OR_AVERAGE_Z_MODE
.In
FME_CLOSE_3D_AVERAGE_MODE
, the curve is closed by modifying the current start and end points of the curve to be the average of the two points.In
FME_CLOSE_3D_EXTEND_MODE
, this method will add a straight segment from the end point to the start point to force the curve closed.In
FME_CLOSE_3D_EXTEND_OR_AVERAGE_Z_MODE
, the curve is closed using the AVERAGE mode if the start and end points only differ in Z. Otherwise, the EXTEND MODE is used.
- Parameters:
- Return type:
FMECurve or None
- Returns:
The closed curve. Note: This method returns a terminal geometry type of the
FMECurve
; i.e. one of the leaf classes in theFMECurve
inheritance graph. For example, aFMEPath
is returned if the geometry truly is a path.- Raises:
FMEException – An exception is raised if an error occurred.
- convertToLine(curve)
Returns a line that is created from the given curve. If the curve is a
FMELine
, it will be returned. If it is aFMEArc
, it will be stroked to a line. If the curve is aFMEPath
, all segments will be returned as their line representations. If the given curve isNone
, nothing will be done. This throws an exception if there is an error.- Parameters:
curve (FMECurve or None) – The curve to convert to a line.
- Return type:
FMELine or None
- Returns:
The line representing the curve.
- Raises:
FMEException – An exception is raised if an error occurred.
- createXMLFromGeometry()
createXMLFromGeometry(geom),
This routine returns an XML definition according to the geometry passed in.
- Parameters:
geom (FMEGeometry) – The geometry to create the XML definition from.
- Return type:
- Returns:
The geometry definition.
- extendToCurve(destinationCurve, sourceCurve)
Extends the source curve to the destination curve. If one curve is a line or a path with a line at its end, and the other curve is a line or a path with a line at its start, the matching lines will be merged, as long as both share the same geometry attributes. If the curves do not have touching end points, a line will be created to attach them. Appending an arc or appending to an arc will result in a path. If the source curve is
None
, nothing will be done andNone
will be returned. If the destination curve isNone
, it will be set to the source curve.- Parameters:
- Return type:
FMECurve or None
- Returns:
The extended curve. Note: This method returns a terminal geometry type of the
FMECurve
; i.e. one of the leaf classes in theFMECurve
inheritance graph. For example, aFMEPath
is returned if the geometry truly is a path.- Raises:
FMEException – An exception is raised if an error occurred.
- extendToPoint(curve, point)
This method will extend the given curve to the given point. This method will add a straight segment from the curve’s end point to the new point. The returned geometry may or may not be the original object that was passed in. If a different object is returned, the original object will be destroyed. If the point is
None
, nothing will be done andNone
will be returned. If the curve isNone
, but the point is valid, a line will be returned that has one point.- Parameters:
- Return type:
FMECurve or None
- Returns:
The extended curve. Note: This method returns a terminal geometry type of the
FMECurve
; i.e. one of the leaf classes in theFMECurve
inheritance graph. For example, aFMELine
is returned if the geometry truly is a line.- Raises:
FMEException – An exception is raised if an error occurred.
- extractTextLocation(text)
This routine consumes the text passed in and returns the
IFMEGeometry
that was its location.- Parameters:
text (FMEText) – The text whose location to extract.
- Return type:
- Returns:
The geometry at the text’s location. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMELine
is returned if the geometry truly is a line.- Raises:
FMEException – An exception is raised if an error occurred.
- force2D(geometry)
This routine forces the geometry to 2D. If the geometry is surface or solid, it will become 2D polygons, or wire-frame if the surface is vertical.
- Parameters:
geometry (FMEGeometry) – The geometry to force 2D.
- Return type:
- Returns:
The geometry in 2D. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMELine
is returned if the geometry truly is a line.- Raises:
FMEException – An exception is raised if an error occurred.
- getPartCount(geometry, recursive, splitDonuts, splitPaths)
Return the number of parts in the geometry. For multis and aggregates, this is the number of parts, and for paths, the number of segments; otherwise it is one. If recursive is
True
, then aggregates’ parts will be counted recursively.- Parameters:
geometry (FMEGeometry) – aggregate or multis whose parts to count.
recursive (bool) – Boolean indicating whether or not to count recursively.
splitDonuts (bool) – Boolean indicating whether or not to split donuts.
splitPaths (bool) – Boolean indictaing whether or to split paths.
- Return type:
- Returns:
The number of parts in the geometry.
- join(firstGeom, secondGeom, aggregatable)
This routine joins two geometries together. Options applying to joining will affect the result. Both geometries will be taken ownership if the result is successful. If the joining of two geometries doesn’t make any sense, put them into an aggregate. For example: one ellipse and one line
- Parameters:
firstGeom (FMEGeometry) – The first geometry to join.
secondGeom (FMEGeometry) – The second geometry to join.
aggregatable (bool) – Boolean indictaing whether or not to combine the two geometries into an aggregate.
- Return type:
- Returns:
The combined geometries. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMELine
is returned if the geometry truly is a line.- Raises:
FMEException – An exception is raised if an error occurred.
- makeDonuts()
makeDonuts(multiArea),
This routine takes in a MultiArea, extracts simple areas from it, and creates donuts out of them. The resulting donuts and simple areas, if any, are returned. The returned MultiArea may contain a mixture of donuts and simple areas.
- Parameters:
multiArea (FMEMultiArea) – The multi area to extract the donuts from.
- Return type:
- Returns:
The donuts and simple areas extracted form the multi area. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMESimpleArea
is returned if the geometry truly is a simple area.- Raises:
FMEException – An exception is raised if an error occurred.
- matrixTransform(geometry, m11, m12, m13, m14, m21, m22, m23, m24, m31, m32, m33, m34)
This method performs a 3D matrix transformation on the geometry passed in. The order in which parameters are passed in is important and it should be row wise e.g. for a matrix the order of the parameters should be m11, m12, m13, m14, m21, m22, m23, m24, m31, m32, m33, m34.
|m11 m12 m13 m14|
|m21 m22 m23 m24|
|m31 m32 m33 m34|
After doing the transformation, geometry is replaced with the transformed geometry if the method is successful. Otherwise, the matrix transform has failed and a
FMEException
is thrown.- Parameters:
geometry (FMEGeometry) – The geometry to apply the transformation Matrix to.
- Return type:
- Returns:
The transformed geometry. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMEAggregate
is returned if the geometry truly is a aggregate.- Raises:
FMEException – An exception is raised if an error occurred.
- offset(geometry, point)
Offsets the geometry by the coords specified by ‘point’. The returned geometry may or may not be the original object that was passed in. If a different object is returned, the original object will be destroyed.
- Parameters:
geometry (FMEGeometry) – The geometry to offset by point.
point (FMEPoint) – The point to offset the geometry by.
- Return type:
- Returns:
The geometry offset by point. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMEPolygon
is returned if the geometry truly is a polygon.- Raises:
FMEException – An exception is raised if an error occurred.
- refineArea(area, refineType)
See the documentation for
refineGeometry()
. Bitmask elements are defined in Refine Area Type.- Parameters:
area (FMEArea) – The area to refine.
refineType (int) – One of:
FME_RA_PATH_CONCAT_LINES
,FME_RA_PATH_EXTRACT_SINGLE_LINES
,FME_RA_PATH_EXTRACT_SINGLE_ARCS
,FME_RA_ARC_STANDARDIZE
,FME_RA_ARC_STROKE
,FME_RA_AREA_STANDARDIZE
,FME_RA_LINE_REMOVE_DUPLICATE_COORDS
, orFME_RA_LINE_REMOVE_DUPLICATE_COORDS_Z
.
- Return type:
- Returns:
The refined area. Note: This method returns a terminal geometry type of the
FMEArea
; i.e. one of the leaf classes in theFMEArea
inheritance graph. For example, aFMEPolygon
is returned if the geometry truly is a polygon.- Raises:
FMEException – An exception is raised if an error occurred.
- refineCurve(curve, refineType)
See the documentation for
refineGeometry()
. Bitmask elements are defined in Refine Curve Type.- Parameters:
curve (FMECurve) – The curve to refine.
refineType (int) – One of:
FME_RC_PATH_CONCAT_LINES
,FME_RC_PATH_EXTRACT_SINGLE_LINES
,FME_RC_PATH_EXTRACT_SINGLE_ARCS
,FME_RC_ARC_STANDARDIZE
,FME_RC_ARC_STROKE
,FME_RC_LINE_REMOVE_DUPLICATE_COORDS
, orFME_RC_LINE_REMOVE_DUPLICATE_COORDS_Z
.
- Return type:
- Returns:
The refined curve. Note: This method returns a terminal geometry type of the
FMECurve
; i.e. one of the leaf classes in theFMECurve
inheritance graph. For example, aFMELine
is returned if the geometry truly is a line.- Raises:
FMEException – An exception is raised if an error occurred.
- refineGeometry(geometry, refineType)
This routine offers a number of options to refine geometries. The options are defined as a bitmask whose components are defined in Refine Geometry Type. Options applying to curves will affect area boundaries.
FME_RG_AGG_CONVERT_HOMOGENEOUS_TO_MULTI
: Any aggregates that could be represented as multis will have their parts removed and a new multi will be the result.FME_RG_AGG_OR_MULTI_RECURSE
: Elements of aggregates and multis will also be refined according to the same parameters.FME_RG_AGG_OR_MULTI_EXTRACT_SINGLETONS
: Aggregates or multis with only one element will have it removed and returned.FME_RG_AREA_STANDARDIZE
: Donuts with no holes will become polygons or ellipses, and polygons with arc boundaries will become ellipses.FME_RG_ARC_STROKE
: Arcs will be converted to lines.FME_RG_ARC_STANDARDIZE
: Center-point arcs will have their parameters adjusted so that start angle is in [0, 360) and rotation is in [0, 90].FME_RG_PATH_EXTRACT_SINGLE_ARCS
: Paths containing one arc (or other non-line segment) and no lines will be replaced by that segment.FME_RG_PATH_EXTRACT_SINGLE_LINES
: Paths containing one line and no arcs will be replaced by that line.FME_RG_PATH_CONCAT_LINES
: Consecutive line parts in a path will be combined into single lines, as long as they contain identical geometry attributes.FME_RG_LINE_REMOVE_DUPLICATE_COORDS
: Consecutive duplicate coordinates are removed. Arcs are unmodified. Only x and y values in the coordinates are used to detect duplicates.FME_RG_LINE_REMOVE_DUPLICATE_COORDS_Z
: Consecutive duplicate coordinates are removed. Arcs are unmodified. x, y, and z values in the coordinates are used to detect duplicates.FME_RG_3D_CONVERT_TO_WIREFRAME
: 3D surfaces and solids will be converted into wire-frames.FME_RG_RASTER_CONVERT_TO_POLYGON
: Rasters will be replaced with their polygon boundaries.FME_RG_3D_CONVERT_TO_POLYGON
: 3D surfaces and solids will be converted into areas.FME_RG_3D_CONVERT_TO_FACE
: 3D surfaces and solids will be converted into faces.FME_RG_3D_REMOVE_VERTICAL_SEGMENTS
: Any vertical segments that exist in three dimensional curves.FME_RG_3D_CONVERT_VERTICAL_FACES_TO_WIREFRAME
: Convert any vertical faces that exist in 3D surfaces and solids to wire-frames.FME_RG_REMOVE_NULLS
: recursively deletes anyFMENull
geometries. Container geometries such as aggregates will become empty if all they contained wasFMENull
. If the user passes in aFMENull
, aFMENull
will be returned.FME_RG_3D_AUTO_FRONT_SIDED_SURFACE
: will modify a surface so that it is front-sided, where the side selected favors the side with a non-default appearance or in the case of two non-default appearances, the side with a texture reference.
- Parameters:
geometry (FMEGeometry) – The geometry to refine.
refineType (int) – One of the Refine Geometry Types.
- Return type:
- Returns:
The geometry to refine. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMEPolygon
is returned if the geometry truly is a polygon.- Raises:
FMEException – An exception is raised if an error occurred.
- rotate2D(geometry, center, angle)
The angle is CCW up from the horizontal and is measured in degrees. The returned geometry may or may not be the original object that was passed in. If a different object is returned, the original object will be destroyed. If an error occurs an exception is thrown.
- Parameters:
geometry (FMEGeometry) – The geometry to rotate.
center (FMEPoint) – The point to rotate the geometry around.
angle (float) – The angle to rotate the geometry by.
- Return type:
- Returns:
The rotated geometry. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMESimpleArea
is returned if the geometry truly is a simple area.- Raises:
FMEException – An exception is raised if an error occurred.
- scale(geometry, xscale, yscale, zscale)
The ‘zscale’ is ignored if geometry is 2D. The returned geometry may or may not be the original object that was passed in. If a different object is returned, the original object will be destroyed. If an error occurs an exception is thrown.
- Parameters:
geometry (FMEGeometry) – The geometry to scale.
xscale (float) – The x scale factor.
yscale (float) – The y scale factor.
zscale (float) – The z scale factor.
- Return type:
- Returns:
The scaled geometry. Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMESimpleArea
is returned if the geometry truly is a simple area.- Raises:
FMEException – An exception is raised if an error occurred.
- setArcPrimaryRadius(arc, primRadius)
This method is deprecated as of FME 2022.1. Use
FMEArc.setPrimaryRadius
instead.The zscale is ignored if geometry is 2D. The returned geometry may or may not be the original object that was passed in. If a different object is returned, the original object will be destroyed. If an error occurs an exception is thrown.
- Parameters:
- Return type:
- Returns:
The arc after the primary radius is set.
- Raises:
FMEException – An exception is raised if an error occurred.
- setArcRotation(arc, rotation)
This method is deprecated as of FME 2022.1. Use
FMEArc.setRotation
instead.Set the rotation on an arc object. All angles are CCW up from the horizontal and are measured in degrees. If the underlying arc is stored by bulge or by 3 points, it will become an arc by center point.
- Parameters:
- Return type:
- Returns:
The arc after setting the rotation.
- Raises:
FMEException – An exception is raised if an error occurred.
- setArcSecondaryRadius(arc, secRadius)
This method is deprecated as of FME 2022.1. Use
FMEArc.setSecondaryRadius`
instead.Sets the secondary radius of the arc. If the underlying arc is stored by bulge or by 3 points, it will become an arc by center point.
- Parameters:
- Return type:
- Returns:
The arc after the secondary radius is set.
- Raises:
FMEException – An exception is raised if an error occurred.
- setArcSweepAngle(arc, angle)
This method is deprecated as of FME 2022.1. Use
FMEArc.setSweepAngle
instead.Sets the sweep angle of the arc. If the angle is a bulge arc, it is turned as an arc by center point to support sweep angle of 360.
- Parameters:
- Return type:
- Returns:
The arc after setting the sweep angle.
- Raises:
FMEException – An exception is raised if an error occurred.
- splitDoubleSidedSurface()
splitDoubleSidedSurface(doubleSidedSurface),
Takes a double-sided surface and splits it up into two single-sided surfaces of the same type. If the passed in geometry is actually single-sided, then
None
is returned for the side that doesn’t exist. An error is returned when the function encounters a problem trying to split up the surface.- Parameters:
doubleSidedSurface (FMESurface) – The surface to split up.
- Return type:
- Returns:
A List of the front and back side of doubleSidedSurface, in the form [frontSideSurface, backSideSurface].
- Raises:
FMEException – An exception is raised if an error occurred.
- triangulateToSurface()
triangulateToSurface(geometry),
This routine will return a
FMEGeometry
object with triangle faces. This feature can support all geometries except forFMEAggregate
,FMECSGSolid
,FMECompositeSolid
andFMEMultiSolid
. The outputFMEGeometry
is neverNone
. The resultingFMEGeometry
is not guaranteed to contain only triangles, so it is the user’s responsiblity to check this. The geometry type of the returnedFMEGeometry
object depends on the type of input geometry:If the input is a
FMETriangleStrip
,FMETriangleFan
, orFMEFace
that is already triangular, the resultingFMEGeometry
is a copy of the input geometry and hence has the same geometry type as the input.If the input is a sliver face, the resulting
FMEGeometry
is a copy of the original geometry.If the input is a non-triangular and non-sliver Face, non-triangular Area, or RectangleFace, the resulting
FMEGeometry
is aFMECompositeSurface
, whose components are triangular Faces. Otherwise the component is aFMECompositeSurface
consisting of triangular Faces.If the input is a CompositeSurface, the resulting
FMEGeometry
is aFMECompositeSurface
. The type of each component in the resultingFMECompositeSurface
is determined as described above.If the input is a MultiSurface, the resulting
FMEGeometry
is aFMEMultiSurface
. The type of each component in the resultingFMEMultiSurface
is determined as described above.If the input is a Box, Extrusion, or BRepSolid, the resulting
FMEGeometry
is aFMEBRepSolid
.If the input is a MultiArea, the resulting
IFMEGeometry
is aFMEMultiSurface
. The type of each component in the resultingFMEMultiSurface
is determined as described above.If the input is a Mesh, the resulting
FMEGeometry
is aFMEMultiSurface
.
For the case of
FMEArea
, the triangulation will occur with respect to the x- and y-coordinates, the z values are ignored, yet they will be retained in the output. If there are any errors an exception will be thrown.
- Parameters:
geometry (FMEGeometry) – The geometry to triangulate to the surface.
- Return type:
- Returns:
Note: This method returns a terminal geometry type of the
FMEGeometry
; i.e. one of the leaf classes in theFMEGeometry
inheritance graph. For example, aFMETriangleStrip
is returned if the geometry truly is a triangle strip.- Raises:
FMEException – An exception is raised if an error occurred.