fmeobjects.FMEBandProperties
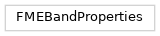
|
Evaluates whether two band properties are identical. |
Returns bit depth of the band. |
|
Returns the interpretation of the band. |
|
Returns the name of the band. |
|
Returns the number of columns in the tile size of the band. |
|
Returns the number of rows in the tile size of the band. |
|
Returns tile type representing the type of data access supported by this band. |
|
Evaluates whether the band properties have been modified from their baseline state. |
|
Sets the interpretation of the band. |
|
|
Sets whether band properties have been modified from baseline state. |
Sets name of the band. |
|
|
Sets the number of columns per tile in the raster |
|
Sets the number of rows per tile in the raster. |
|
Sets the tile type that can most efficiently support data access. |
- class FMEBandProperties
FME Band Properties Class
Create an instance of a
FMEBandProperties
object.init(nameString, interpretation, tileType, numTileRows, numTileColumns)
Creates a band property with the given properties.
- Parameters:
FME_TILE_TYPE_FIXED
: Tile requests made to a band must be equal to the band’s tile size.FME_TILE_TYPE_FIXED_MULTIPLE
: Tile requests made to a band must be a multiple of the band’s tile size.FME_TILE_TYPE_FLEXIBLE
: The band tile size is a suggestion only, and the band can handle any arbitrary tile size.
- Parameters:
numTileRows – Number of rows per tile in the raster.
numTileColumns – Number of columns per tile in the raster.
- Return type:
- Returns:
An instance of a BandProperties object.
init(bandProperties)
Create a copy of the passed in bandProperties object.
- Parameters:
bandProperties (FMEBandProperties) – The bandProperties object to create a copy of.
- Return type:
- Returns:
An instance of a BandProperties object.
- __init__(*args, **kwargs)
- equals(bandPropertyObj)
Evaluates whether two band properties are identical.
- Parameters:
bandPropertyObj (FMEBandProperties) – The band property to compare to.
- Return type:
- Returns:
True
if the two properties have the same value.
- getInterpretation()
Returns the interpretation of the band.
Returns one of:
- Return type:
- Returns:
The interpretation of the band.
- getName()
Returns the name of the band.
- getNumTileCols()
Returns the number of columns in the tile size of the band. That is, this represents the most efficient tile size for data access. Note that a band can return data for any tile size (i.e.
FMEBand.getTile
will accept any tile), but using a tile with this size may have better performance or lower memory usage.- Return type:
- Returns:
The number of columns per tile.
- getNumTileRows()
Returns the number of rows in the tile size of the band. That is, this represents the most efficient tile size for data access. Note that a band can return data for any tile size (i.e.
FMEBand.getTile
will accept any tile), but using a tile with this size may have better performance or lower memory usage.- Return type:
- Returns:
The number of rows per tile.
- getTileType()
Returns tile type representing the type of data access supported by this band. Possible values:
FME_TILE_TYPE_FIXED
: Tile requests made to a band must be equal to the band’s tile size.FME_TILE_TYPE_FIXED_MULTIPLE
: Tile requests made to a band must be a multiple of the band’s tile size.FME_TILE_TYPE_FLEXIBLE
: The band tile size is a suggestion only, and the band can handle any arbitrary tile size. WhengetTileType()
returnsFME_TILE_TYPE_FIXED
, the band tile size (fromgetNumTileRows()
andgetNumTileCols()
) is a requirement. That is, only tiles of the specified size can be properly filled. WhengetTileType()
returnsFME_TILE_TYPE_FLEXIBLE
, the band tile size is only a suggestion, and it implies the most efficient tile size for data access.
Note that this value is only relevant for readers. That is, when creating a reader, this value should be specified depending on how
FMEBandTilePopulator
is implemented. On the other hand, a consumer of data (e.g. a writer) can always request data in whatever size is desired, regardless of this value. FME will reconcile the writer request size with the reader’s supported tile size.- Return type:
- Returns:
Type of the tile.
- hasBeenModified()
Evaluates whether the band properties have been modified from their baseline state.
- setInterpretation(interpretation)
Sets the interpretation of the band.
- Parameters:
interpretation (int) –
Interpretation of the band. Choose one of:
- Return type:
None
- setModified(modified)
Sets whether band properties have been modified from baseline state.
Note that
hasBeenModified
is initially set to False.
- setName(name)
Sets name of the band.
- Parameters:
name (str) – The name of the band.
- Return type:
None
- setNumTileCols(numCols)
Sets the number of columns per tile in the raster
- Parameters:
numCols (int) – Number of columns per tile in the raster. Number must be positive number, not exceeding the total number of columns.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setNumTileRows(numRows)
Sets the number of rows per tile in the raster.
- Parameters:
numRows (int) – Number of rows per tile in the raster. Number must be positive number, not exceeding the total number of rows.
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.
- setTileType(tileType)
Sets the tile type that can most efficiently support data access.
- Parameters:
tileType (int) – Possible values:
FME_TILE_TYPE_FIXED
: Tile requests made to a band must be equal to the band’s tile size.FME_TILE_TYPE_FIXED_MULTIPLE
: Tile requests made to a band must be a multiple of the band’s tile size.FME_TILE_TYPE_FLEXIBLE
: The band tile size is a suggestion only, and the band can handle any arbitrary tile size.
- Return type:
None