fmeobjects.FMESpatialIndex
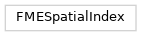
This method returns the bounding box of all the data stored in the spatial index. |
|
|
This method writes out the spatial index, and closes any files the spatial index has open. |
This method returns a count of features that depends on what mode the index is currently in. |
|
This method fetches the next feature from the current query. |
|
This method opens the spatial index using the mode specified and file name specified during creation. |
|
This method retrieves all features currently stored in the index. |
|
|
This method performs a query to determine which features in the index have an area that intersects the query feature. |
queryClosest(xCoord, yCoord, maxDist), |
|
queryClosestByFeatureType(xCoord, yCoord, maxDist, featTypes), |
|
|
This method performs a query to determine which features in the index share a common line segment with the query feature. |
|
This method performs a query to determine which features in the index have a common vertex with the query feature. |
This method performs a query to determine which features in the index share either a common vertex or a common segment with the query feature. |
|
|
This method performs a query to determine which features in the index are contained by the query feature. |
|
This method performs a query to determine which features in the index contain the query feature. |
|
This method performs a query to determine which features in the index have envelopes that intersect the envelope of the query feature. |
This method performs a query to determine which features in the index have envelopes that intersect the envelope of the query feature. |
|
|
This method performs a query to determine the farthest feature to the passed in point that is within the search distance. |
|
This method performs a query to determine which features in the index are geometrically identical to the query feature. |
|
This method performs a query to determine which features in the index intersect with the query feature. |
|
This method performs a query to determine the total number of features that are within the specified maxDist from the x and y coordinate. |
|
This method sets the maximum file size (in bytes) for each file used by the index. |
|
This method stores the feature in the spatial index. |
This method stores a feature using the specified rectangles for the index rather than the extent of the feature itself. |
- class FMESpatialIndex
FME Spatial Index Class
init(fileName, accessMode, directives)
Create a spatial index object which stores the features in a file so as not to keep all features in the index in memory. This enables users of FME Objects to work with large datasets in an efficient manner.
- Parameters:
fileName (str) – Indicates which spatial index to open for reading or the location of where the new spatial index will be created. It’s the full path of the file without extension. Each spatial index will have two files named:
<fileName>.ffs: The actual feature coordinates and attributes.
<fileName>.fsi: The spatial index also stored on disk.
- Parameters:
accessMode – Indicates the intended use of the spatial index. Valid values are restricted to:
- “READ”: Open an existing spatial index and extract data from it. Data cannot
be written in this mode.
- “WRITE”: Create a new spatial index. In this case data can be written only.
Features cannot be updated or deleted however.
- Parameters:
directives – (Optional) Directives are key/value pairs that can be passed to the creation of the spatial index. If no directives are to be specified then an empty dict or list may be specified. The supported directives are:
kFME_SIPassphrase
: The spatial index passphrase allows users torestrict access to spatial index files. If a passphrase directive is used when a spatial index is created, this passphrase must also be supplied in future attempts to open the spatial index. A spatial index cannot be opened if it was created with a passphrase and an incorrect passphrase is given when reading is attempted. If this directive is not provided, no passphrase is applied to the spatial index and all future attempts to read the spatial index also do not need to specify a passphrase. Passphrases may be any character string of any length or content.
kFME_SISerializeRasterData
: Specifies how to treat raster features.If the value is “yes”, then any raster features stored in the spatial index will be written to disk. This will take a longer time, but is suitable if the spatial index is intended to be persistent. If the value is “no”, then raster features stored in the spatial index will be kept in memory as long as the spatial index exists, but will not be written to disk. This is faster, and is suitable when the spatial index is only intended to be a temporary construct.
- Return type:
- Returns:
An instance of a Spatial Index object.
- __init__(*args, **kwargs)
- boundingBox()
This method returns the bounding box of all the data stored in the spatial index.
- Return type:
- Returns:
The bounding box of the data in the form ((minx, miny), (maxx, maxy)).
- Raises:
FMEException – An FMEException is raised if there was an error.
- close(deleteIndex)
This method writes out the spatial index, and closes any files the spatial index has open.
- Parameters:
deleteIndex (bool) – If
True
, then it will also delete any files being used by the spatial index.- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- entries()
This method returns a count of features that depends on what mode the index is currently in.
- Read Mode: this method returns the numbers of entries left for the current
query.
Write Mode: this method returns the numbers of features stored in the index.
- Return type:
- Returns:
The current count of features.
- fetch()
This method fetches the next feature from the current query. If the current query has no further results to return, then
None
is returned.- Return type:
FMEFeature or None
- Returns:
The next feature from the current query or None if there are no further results.
- Raises:
FMEException – An FMEException is raised if there was an error.
- open()
This method opens the spatial index using the mode specified and file name specified during creation.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryAll()
This method retrieves all features currently stored in the index.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryAreaIntersect(queryFeature)
This method performs a query to determine which features in the index have an area that intersects the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryClosest()
queryClosest(xCoord, yCoord, maxDist),
This method performs a query to determine the closest feature to the passed in point that is within the search distance.
- Parameters:
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryClosestByFeatureType()
queryClosestByFeatureType(xCoord, yCoord, maxDist, featTypes),
This method performs a query to determine the closest feature to the passed in point that is within the search distance. Only the specified feature types are searched.
- Parameters:
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryCommonSegment(queryFeature)
This method performs a query to determine which features in the index share a common line segment with the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryCommonVertex(queryFeature)
This method performs a query to determine which features in the index have a common vertex with the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryCommonVertexOrSegment(queryFeature)
This method performs a query to determine which features in the index share either a common vertex or a common segment with the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryContainedBy(queryFeature)
This method performs a query to determine which features in the index are contained by the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryContains(queryFeature)
This method performs a query to determine which features in the index contain the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryEnvelope(queryFeature)
This method performs a query to determine which features in the index have envelopes that intersect the envelope of the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryEnvelopeByFeatureType(queryFeature, featTypes)
This method performs a query to determine which features in the index have envelopes that intersect the envelope of the query feature. Only the specified feature types are searched.
- Parameters:
queryFeature (FMEFeature) – The query feature.
featTypes (list[str]) – The list of feature types to be searched.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryFarthest(xCoord, yCoord, maxDist)
This method performs a query to determine the farthest feature to the passed in point that is within the search distance.
- Parameters:
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryIdentical(queryFeature)
This method performs a query to determine which features in the index are geometrically identical to the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryLinesCross(queryFeature)
This method performs a query to determine which features in the index intersect with the query feature. I.e. the boundary of the returned features crosses the boundary of the query feature.
- Parameters:
queryFeature (FMEFeature) – The query feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- queryTotal(xCoord, yCoord, maxDist)
This method performs a query to determine the total number of features that are within the specified maxDist from the x and y coordinate.
- Parameters:
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- setMaxFileSize(maxFileBytes)
This method sets the maximum file size (in bytes) for each file used by the index. Caveats:
Multiple files will be used by the index if necessary.
- Features are not split across files, and therefore this maximum is not
guaranteed to be precise.
- Parameters:
maxFileBytes (int) – The maximum file size in bytes for each file.
- Return type:
None
- store(feature)
This method stores the feature in the spatial index.
- Parameters:
feature (FMEFeature) – The feature to store in the spatial index.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- storeUsingIndexRects(feature, indexRects)
This method stores a feature using the specified rectangles for the index rather than the extent of the feature itself.
- Parameters:
feature (FMEFeature) – The feature to store in the spatial index.
indexRects (list[tuple[tuple[float]]]) – The list of rectangles used for the index. Each rectangle is represented by a tuple of tuple of floats in the form ((minx, miny), (maxx, maxy)).
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.