fmeobjects.FMEFactoryPipeline
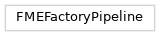
|
This method is used to add a set of factories to the end of the factory pipeline. |
|
This method is used to add a new factory to the end of the factory pipeline. |
This method is used to indicate that no more features will be entering the pipeline. |
|
This method is used to specify configuration lines for functions such as @Lookup, @Relate, and @Sql, which require configuration information to operate. |
|
If |
|
This method is used to fetch a feature ready for output from the pipeline. |
|
processFeature(feature), |
|
setCallback(function), |
- class FMEFactoryPipeline
FME Factory Pipeline Class
Creates a new factory pipeline object.
init(name)
Constructs a factory pipeline object.
- Parameters:
name (str) – The name of the factory pipeline.
- Return type:
- Returns:
This method returns a new factory pipeline object.
init(name, directives)
Constructs a factory pipeline object.
- Parameters:
name (str) – The name of the factory pipeline.
directives –
Additional directives to specify. Directives are of the form of a directive name as an entry in the list, with the next entry having the value for the directive. An empty list of str can be used to specify that no directives are present. The supported directives are :
<–macro_name> Any directive that begins with two dashes is considered to be a macro name that will be set for use within the pipeline. For example, if a directive name ‘–MySetting’ with a value of ‘Yes’ is given, then the macro ‘MySetting’ will be given a value of ‘Yes’, for use within the pipeline.
- Return type:
- Returns:
This method returns a new factory pipeline object.
- __init__(*args, **kwargs)
- addFactories(factoryDefFile)
This method is used to add a set of factories to the end of the factory pipeline. If unsuccessful, this method throws an exception.
- Parameters:
factoryDefFile (str) – Specifies the name of a file containing the factory definitions.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- addFactory(factoryDef, delimiter)
This method is used to add a new factory to the end of the factory pipeline. If ‘factoryDef’ is a list of str the factory to be added is specified as a set of tokens; one token for every whitespace-delimited str in the factory definition. Each token is represented as one entry in the ‘factoryDef’ parameter. For example, the PolygonFactory show below is be specified using 20 tokens:
FACTORY_DEF
* PolygonFactoryFACTORY_NAME
SamplePolygonINPUT FEATURE_TYPE
*GROUP_BY
someAttrVERTEX_NODED
REMOVE_CORRIDORS
OUTPUT POLYGON FEATURE_TYPE
polyOUTPUT LINE FEATURE_TYPE
line
If ‘factoryDef’ is a str, it specifies the factory to be added as an ASCII character str. The ‘delimiter’ parameter specifies the str to be used as delimiter when parsing the ‘factoryDef’ string for tokens.
- Parameters:
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- allDone()
This method is used to indicate that no more features will be entering the pipeline. Any factories in the pipeline that are still holding features will release them when this method is called. This method can only be called once on per pipeline.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- configureFunction(functionConfig)
This method is used to specify configuration lines for functions such as @Lookup, @Relate, and @Sql, which require configuration information to operate. Refer to the FME Functions and Factories manual for detailed information.
- Parameters:
functionConfig (list[str]) – The configuration information for a function.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- getErrorMessage()
If
allDone()
returns an error, the whole message produced can be retrieved with this method.
- getOutputFeature()
This method is used to fetch a feature ready for output from the pipeline. If no feature is ready for output, an exception is thrown. This method should not be used if the
setCallback()
method has been called on the pipeline.- Return type:
FMEFeature or None
- Returns:
The feature ready for output or
None
if all the features have been outputted.- Raises:
FMEException – An FMEException is raised if there was an error.
- processFeature()
processFeature(feature),
This method is used to insert a feature into the pipeline for processing. On output the feature is returned void of all attributes and geometry.
- Parameters:
feature (FMEFeature) – The feature to insert into the pipeline.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- setCallback()
setCallback(function),
This method is used to specify the name of a function that will be called by the pipeline when a feature is ready for output. If a callback function is specified, features must not be retrieved using the
getOutputFeature()
method.- Parameters:
function (Callable) – The function to provide FME for use as a callback function. The passed in function must take in a
FMEFeature
as the parameter and returnTrue
on success, andFalse
upon failure.- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.