fmeobjects.FMERasterTools
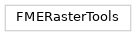
|
Adds a band tile cache to a band. |
Performs an affine transformation on a raster. |
|
|
Performs rotation on a raster to remove the rotation properties but maintain a similar visual image. |
|
Calculates the aspect (direction of slope) for each selected band of a raster. |
|
Performs a calculation of the distance between a vector line and a raster DEM. |
|
Calculate the slope (maximum rate of change in z) for each selected band of a raster. |
|
Sets a checkpoint in the raster processing which forces previous processing to occur immediately and saves the current state to disk once complete. |
|
Performs clipping on a raster. |
|
Clips a raster with a set of clipper geometries. |
Converts data type of a band. |
|
|
Converts data type of a raster. |
Converts data type of a palette. |
|
|
Creates a new band to represent the grouped single nodata value of all selected bands on the raster. |
Replaces the selected band(s) on the raster by a new band with a new palette. |
|
|
Generates a shaded relief effect, useful for visualizing terrain. |
|
Mosaics multiple rasters into one raster. |
|
Performs offsetting on a raster. |
Removes nodata value on the band. |
|
|
Replaces a range of value in the source raster with a new single value. |
Replaces ranges of values in the source raster with new values. |
|
|
Performs coordinate system reprojection on a raster. |
|
Performs resampling (both upsampling and downsampling) on a raster using number of rows and columns. |
|
Performs resampling (both upsampling and downsampling) on a raster using 'xSpacing' and 'ySpacing'. |
|
Resolves the values in a raster's bands to the values in the lookup palettes associated with each band. |
|
Adjusts the existing rotation angle on a raster by the specified 'rotationAngle'. |
|
Performs scaling on a raster. |
Sets the nodata value on the band. |
|
|
Performs clipping of a raster using pixel bounds rather than ground coordinates. |
- class FMERasterTools
FME Raster Tools Class
init()
This class provides the ability to modify FME Raster.
- __init__(*args, **kwargs)
- addBandTileCache(band, parms)
Adds a band tile cache to a band.
Adding a cache may improve performance in cases where a band will be read multiple times. In such cases, data read from the band will be cached, so that subsequent callers may have their
FMEBand.getTile()
requests filled without performing redundant processing.Note that a cache will not by default accumulate tiles. When created it is in an “undecided” state. In this state, it will accumulate tiles only if multiple instances of it exist(e.g.a clone is made after adding the cache). In order to force a cache to accumulate tiles, call
FMEBand.enableBandCache()
after calling this method.Adding a cache will be most useful when consumers request data in a tiled - BSQ interleaving. That is, when iterating over tiles first, then bands.
- Parameters:
- Return type:
- Returns:
The band after adding cache to a band.
- Raises:
FMEException – An exception is raised if an error occurred.
- affineTransform(affineTransformList, raster)
Performs an affine transformation on a raster.
- Parameters:
- Return type:
- Returns:
The raster after performing an affine transformation.
- Raises:
FMEException – An exception is raised if an error occurred.
- applyRotation(interpolation, raster)
Performs rotation on a raster to remove the rotation properties but maintain a similar visual image.
The raster will be rotated by the negative of the existing rotation angle of the input raster so that the resultant raster will have a rotation angle of zero.
- Parameters:
interpolation (int) –
Interpolation type of the raster. Choose one of:
FME_INTERPOLATION_NEARESTNEIGHBOR
FME_INTERPOLATION_BILINEAR
FME_INTERPOLATION_BICUBIC
FME_INTERPOLATION_AVERAGE4
FME_INTERPOLATION_AVERAGE16
FME_INTERPOLATION_SOURCE
raster (FMERaster) – Raster object to apply rotation to.
- Return type:
- Returns:
The raster after applying rotation.
- Raises:
FMEException – An exception is raised if an error occurred.
- calculateAspect(raster, parms)
Calculates the aspect (direction of slope) for each selected band of a raster.
Aspect is measured in degrees from 0 to 360, starting clockwise from the north. Each selected input band will be converted to a REAL64 band with output values that represent the aspect. If an input band does not have a nodata value, the output band nodata value will be set to - 1.
- Parameters:
raster (FMERaster) – The raster object where the bands belong.
(Optional) Name-value pair representing additional parameters.
Possible parms are:
kFME_CalculateAspect_interpolateNodata
Yes or No (Default is No)
Whether to calculate values at raster edges and near nodata values. When this is set to No, there will be a one pixel border around the edge of the raster set to the nodata value. Additionally, when any pixel in the 3x3 window used to calculate the aspect value is equal to nodata, the output pixel will also be set to nodata. When this is set to Yes, values around the edge and near nodata values will be estimated by interpolating missing values.
kFME_CalculateAspect_algorithm
kFME_CalculateAspect_algorithm_Horn
- Use Horn’s method for slope/aspect calculation. Better suited to rougher terrain.kFME_CalculateAspect_algorithm_ZevenbergenThorne
- Use Zevenbergen & Thorne’s method for slope/aspect calculation. Better suited to smooth terrain.
- Return type:
- Returns:
The raster after calculating aspect.
- Raises:
FMEException – An exception is raised if an error occurred.
- calculateDEMDistance(line, band)
Performs a calculation of the distance between a vector line and a raster DEM.
The line parameter is the input vector line. Having fewer vertices on the input line will improve the performance of this function but may decrease the accuracy of the data.
The band parameter must be a numeric Digital Elevation Model (DEM) without a palette.
The output band will also be a DEM containing smaller values where the distance from the source DEM elevations is less(darker areas) and larger values where the distance between the source DEM elevations is greater(lighter areas).
The line must be in the same coordinate system as the raster.
- Parameters:
- Return type:
- Returns:
The band after calculating DEM distance.
- Raises:
FMEException – An exception is raised if an error occurred.
- calculateSlope(slopeType, raster, parms)
Calculate the slope (maximum rate of change in z) for each selected band of a raster.
- Parameters:
Possible parms are:
kFME_CalculateSlope_interpolateNodata
Yes or No (Default is No)
Whether to calculate values at raster edges and near nodata values. When this is set to No, there will be a one pixel border around the edge of the raster set to the nodata value. Additionally, when any pixel in the 3x3 window used to calculate the slope value is equal to nodata, the output pixel will also be set to nodata. If the input band does not have a nodata value, the output band nodata value will be set to -1. When this is set to Yes, values around the edge and near nodata values will be estimated by interpolating missing values.
(Default) Use Horn’s method for slope/aspect calculation. Better suited to rougher terrain.
Use Zevenbergen & Thorne’s method for slope/aspect calculation. Better suited to smooth terrain.
- Return type:
- Returns:
The raster after calculating the slope.
- Raises:
FMEException – An exception is raised if an error occurred.
- checkpoint(raster)
Sets a checkpoint in the raster processing which forces previous processing to occur immediately and saves the current state to disk once complete.
Checkpointing a raster may improve performance when working with large rasters, where all the necessary data cannot be cached in memory.
The temporary data file will be deleted when the raster is destroyed.
- Parameters:
raster (FMERaster) – Raster object.
- Return type:
- Returns:
The raster after setting the checkpoint.
- Raises:
FMEException – An exception is raised if an error occurred.
- clip(xMin, yMin, xMax, yMax, raster, parms)
Performs clipping on a raster.
The xMin, yMin, xMax, and yMax parameters specify the extents in ground units to which the raster will be clipped.
If the extents do not intersect the raster, exception will be raised.
If the extents align with or are larger than the raster extents, no processing will occur.
- Parameters:
Possible parms are :
Yes or No (Default is No)
Specifies whether to preserve the extents of the input raster. If No, then the raster will be reduced to the specified extents. If Yes, the raster’s extents will not be modified, and areas outside the specified clip extents but within the input raster’s extents will be filled with nodata.
- Return type:
- Returns:
The raster after clipping.
- Raises:
FMEException – An exception is raised if an error occurred.
- clipGeometry(clippers, candidate, parms)
Clips a raster with a set of clipper geometries.
- Parameters:
clippers (FMEAggregate) – The clippers must be areas.
candidate (FMERaster) – The raster to clip.
parms (dict[str, str]) – (Optional) Name-value pair representing additional parameters.
Possible parms are :
kFME_Clip_cellGeometry
center or boundary. (Default is center)
Specifies how each cell is represented when tested against clippers. If set to center, then each cell is represented by its center point. That is, if the center point of a cell is inside a clipper, that cell is considered to be inside. If set to boundary, then the cell boundary (i.e. the entire cell) is considered. That is, a cell is only considered to be inside when the entire cell is inside a clipper.
kFME_clip_treatBoundaryAsInside
Yes or No. (Default is No)
Specifies whether to consider cells where the center point falls on the clipper boundary as inside. Only applicable when the cell geometry is center.
kFME_clip_treatBoundaryAsOutside
Yes or No. (Default is No)
Specifies whether to consider cells where the center point falls on the clipper boundary as outside. Only applicable when the cell geometry is center.
kFME_clip_createOutside
Yes or No. (Default is No)
Specifies whether to create an additional raster containing the outside cells. That is, if this option is yes, the output aggregate will contain (numClippers+1) geometries, where the last one contains the cells that are not contained by any of the clippers. Specifying no may improve performance.
Yes or No (Default is No)
Specifies whether to preserve the extents of the input raster. If No, then each raster will be reduced to the inside area. If Yes, the raster’s extents will not be modified, and areas outside the specified clip extents but within the input raster’s extents will be filled with nodata.
kFME_Clip_addNodata
Yes or No. (Default is No)
Specifies whether nodata should be added (by introducing an alpha band for RGB rasters, or adding a palette entry for bands with palettes) when “empty” areas are being introduced in a raster (e.g. for a non-rectangular clipper, or for the outside raster).
kFME_Clip_clippedTraitName
Specifies the name of a trait that will be set on each output part, specifying whether that part was clipped or not. That is, the trait will be set to true if the geometry is a subset of the input raster, or false if the geometry is identical to the input raster.
- Return type:
- Returns:
An aggregate containing a raster or null geometry for each clipper, depending on whether the clipper intersects the raster.
- Raises:
FMEException – An exception is raised if an error occurred.
- convertBandInterpretation(interpretation, band, parms)
Converts data type of a band.
Note that this method is similar to
convertInterpretation
with a mode ofFME_REINTERPRET_MODE_BAND
, except that this operates directly on a single band, rather than on all the selected bands of a raster.- Parameters:
interpretation (int) –
Desired interpretation of the raster after processing. Choose one of:
FME_INTERPRETATION_NULL
FME_INTERPRETATION_REAL64
FME_INTERPRETATION_REAL32
FME_INTERPRETATION_UINT64
FME_INTERPRETATION_INT64
FME_INTERPRETATION_UINT32
FME_INTERPRETATION_INT32
FME_INTERPRETATION_UINT16
FME_INTERPRETATION_INT16
FME_INTERPRETATION_UINT8
FME_INTERPRETATION_INT8
FME_INTERPRETATION_GRAY8
FME_INTERPRETATION_GRAY16
FME_INTERPRETATION_RED8
FME_INTERPRETATION_RED16
FME_INTERPRETATION_GREEN8
FME_INTERPRETATION_GREEN16
FME_INTERPRETATION_BLUE8
FME_INTERPRETATION_BLUE16
FME_INTERPRETATION_ALPHA8
FME_INTERPRETATION_ALPHA16
band (FMEBand) – The band object to apply conversion to.
parms (dict[str, str]) – (Optional) The parms parameter contains the information on how to do specific conversions. Same parameters as in
convertInterpretation()
.
- Return type:
- Returns:
The band after converting band interpretation.
- Raises:
FMEException – An exception is raised if an error occurred.
- convertInterpretation(mode, interpretation, raster, parms)
Converts data type of a raster.
There are many assumptions on the input raster and the parameters:
- In RASTER mode
When converting to RGB / RGBA, the input raster must contain exactly 1, 3, or 4 bands.
When converting from 4 bands to RGB while trying to apply alpha, the 4 bands must be RGBA. If they are not, make this conversion in two steps (4 bands to RGBA, then RGBA to RGB).
When averaging multiple bands (converting to a single band), scaling by data values or data type is not permitted. Again, the same behavior can be achieved in a two-step conversion : RGB48 to Gray16, which is then scaled by data type to Gray8 for example.
- In PALETTE mode
All selected bands must have at least one selected palette.
- Parameters:
mode (int) –
Specifies what the conversion will work on, the raster, the bands, or the palettes. The Legacy mode should not be used. Choose one of:
FME_REINTERPRET_MODE_RASTER
FME_REINTERPRET_MODE_BAND
FME_REINTERPRET_MODE_PALETTE
interpretation (int) –
Desired interpretation of a raster after processing. Choose one of:
FME_INTERPRETATION_NULL
FME_INTERPRETATION_REAL64
FME_INTERPRETATION_REAL32
FME_INTERPRETATION_UINT64
FME_INTERPRETATION_INT64
FME_INTERPRETATION_UINT32
FME_INTERPRETATION_INT32
FME_INTERPRETATION_UINT16
FME_INTERPRETATION_INT16
FME_INTERPRETATION_UINT8
FME_INTERPRETATION_INT8
FME_INTERPRETATION_GRAY8
FME_INTERPRETATION_GRAY16
FME_INTERPRETATION_RED8
FME_INTERPRETATION_RED16
FME_INTERPRETATION_GREEN8
FME_INTERPRETATION_GREEN16
FME_INTERPRETATION_BLUE8
FME_INTERPRETATION_BLUE16
FME_INTERPRETATION_ALPHA8
FME_INTERPRETATION_ALPHA16
FME_INTERPRETATION_RGB24
FME_INTERPRETATION_RGBA32
FME_INTERPRETATION_RGB48
FME_INTERPRETATION_RGBA64
FME_INTERPRETATION_STRING
raster (FMERaster) – The raster to apply conversion to.
parms (dict[str, str]) – (Optional) Name-value pair representing additional parameters.
Possible parms are :
kFME_ConvertInterpretation_manyToOneBand
(Default) Averages all given bands into a single one.
kFME_ConvertInterpretation_RGBAToRGB
(Default) Discards the alpha band in RGBA to RGB conversions.
Multiplies all RGB values with their corresponding, normalized alpha value.
kFME_ConvertInterpretation_RGBToRGBA
(Default) Creates a new alpha band which has the maximum value for the data type in all cells.
Creates a new alpha band which has the maximum value for the data type only in data cells. A cell is considered to be nodata when, for each selected band, the value for that cell is equal to that band’s nodata value. If any cell value is not equal to that band’s nodata value, the cell will be considered data. Note that when this option is selected, it is required that all input bands have a nodata value.
kFME_ConvertInterpretation_colorToColor
Uses C-style casts to convert the values.
Uses C-style casts to convert the values, but also validates that the source values fit into the destination type, effectively preventing underflow and overflow; if a source value does not fit, the corresponding destination value will either be set to its type’s minimum or maximum value.
Finds the minimum and maximum values of the source values and uses them to scale the values to the full range of the destination type.
(Default) Scales the source values while preserving all proportions in regard to the source and destination types’ range.
kFME_ConvertInterpretation_colorToNumeric
Same possible values as
kFME_ConvertInterpretation_colorToColor
Defaults to BOUNDED_CAST
kFME_ConvertInterpretation_numericToColor
Same possible values as
kFME_ConvertInterpretation_colorToColor
kFME_ConvertInterpretation_numericToNumeric
Same possible values as convertInterpretation_colorToColor`
kFME_ConvertInterpretation_floatToInteger
(Default) Rounds to floating-point value to the nearest integer.
Gets the next integer which is greater than or equal to the floating-point value.
Gets the next integer which is less than or equal to the floating-point value.
- Return type:
- Returns:
The raster after converting the interpretation.
- Raises:
FMEException – An exception is raised if an error occurred.
- convertPaletteInterpretation(keyInterpretation, valueInterpretation, palette, nodataKey, parms)
Converts data type of a palette.
Note that this method is similar to
convertInterpretation
with a mode ofFME_REINTERPRET_MODE_PALETTE
, except that this operates directly on a single palette, rather than on all the selected bands of a raster.- Parameters:
keyInterpretation (int) –
Specifies the desired interpretation of the palette after processing. Choose one of:
FME_INTERPRETATION_UINT8
FME_INTERPRETATION_UINT16
FME_INTERPRETATION_UINT32
valueInterpretation (int) –
Specifies the desired interpretation of the palette after processing. Choose one of:
FME_INTERPRETATION_NULL
FME_INTERPRETATION_RGB24
FME_INTERPRETATION_RGBA32
FME_INTERPRETATION_RGB48
FME_INTERPRETATION_RGBA64
FME_INTERPRETATION_STRING
palette (FMEPalette) – The palette to apply conversion to.
nodataKey (FMETile) – (Optional) Specifies the nodata value of the palette. It must have the same interpretation as the existing palette.
parms (dict[str, str]) – (Optional) Name-value pair that contains information on how to do specific conversions. Same parameters as in
convertInterpretation()
.
- Return type:
- Returns:
The palette after converting the interpretation.
- Raises:
FMEException – An exception is raised if an error occurred.
- createNodataMask(nodataValue, realDataValue, raster, parms)
Creates a new band to represent the grouped single nodata value of all selected bands on the raster.
A cell is considered to be nodata when, for each selected band, the value for that cell is equal to that band’s nodata value. If any cell value is not equal to that band’s nodata value, the cell will be considered data.
The new band will be appended to the end of the raster. All selected bands are required to have a nodata value. The nodataValue and realDataValue tiles must be the same interpretation. The new band will be created with this interpretation.
- Parameters:
Possible parms are :
kFME_CreateNodataMask_removeNodata
Yes or No (Default is No)
Specifies whether the nodata value should be removed from the selected bands after generating the mask.
- Return type:
- Returns:
The raster after creating nodata mask.
- Raises:
FMEException – An exception is raised if an error occurred.
- generatePalette(interpretation, numPaletteEntries, raster, parms)
Replaces the selected band(s) on the raster by a new band with a new palette.
The palette will have at most ‘numPaletteEntries’ number of entries and the interpretation.
The interpretation must be large enough for the numPaletteEntries. For example, when the interpretation is
FME_INTERPRETATION_UINT8
, then the ‘numPaletteEntries’ must not be larger than 256. If the interpretations of the selected bands do not make up a valid palette value interpretation, exception will be raised.- Parameters:
interpretation (int) –
Interpretation of the palette key. Choose one of:
FME_INTERPRETATION_UINT8
FME_INTERPRETATION_UINT16
FME_INTERPRETATION_UINT32
numPaletteEntries (int) – Number of palette entries.
raster (FMERaster) – Raster object where the bands belong.
parms (dict[str, str]) – (Optional) Name-value pair representing additional parameters. Currently, no additional parameters exist.
- Return type:
- Returns:
The raster after generating palette.
- Raises:
FMEException – An exception is raised if an error occurred.
- hillshade(azimuth, altitude, raster, parms)
Generates a shaded relief effect, useful for visualizing terrain.
Each selected input band will be converted to a GRAY8 band with hillshade values between 0 and 255. Additionally, if <interpolate nodata> is disabled or if the band has a nodata value, an additional ALPHA8 band will be generated, with a value of 0 where the input was nodata and a value of 255 where the input was data.
- Parameters:
azimuth (float) – Angle of the light source, expressed in positive degrees from 0 to 360, measured clockwise from north. The value 315 is typically used to generate shaded maps.
altitude (float) – Altitude angle of the light source above the horizon, expressed in positive degrees, with 0 degrees at the horizon and 90 degrees directly overhead.
raster (FMERaster) – Raster object to apply shading effect to.
parms (dict[str, str]) – (Optional) Name-value pair representing additional parameters.
Possible parms are :
kFME_Hillshade_interpolateNodata
Yes or No (Default is No)
Whether to calculate values at raster edges and near nodata values. When this is set to No, there will be a one pixel border around the edge of the raster set to the nodata value (i.e. the alpha band value will be set to 0). Additionally, when any pixel in the 3x3 window used to calculate the hillshade value is equal to nodata, the output pixel will also be set to nodata. When this is set to Yes, values around the edge and near nodata values will be estimated by interpolating missing values.
(Default) Use Horn’s method for slope/aspect calculation. Better suited to rougher terrain.
Use Zevenbergen & Thorne’s method for slope/aspect calculation. Better suited to smooth terrain.
- Return type:
- Returns:
The raster after applying the shading effect.
- Raises:
FMEException – An exception is raised if an error occurred.
- mosaic(inputRasters, parms)
Mosaics multiple rasters into one raster. There are some assumptions of the inputRasters:
Same coordinate system.
Same number of selected bands.
Same band interpretation in each group of bands to be mosaicked when there is no palette.
Same number of selected palettes, either 0 or 1.
Same band nodata value or NULL when there is no palette.
Same value interpretation in the selected palette.
Same palette nodata value or NULL where there is a palette.
When compositing using alpha, each raster has exactly one alpha band.
When overlapping values is set to average, sum, or composite_using_alpha palettes are not supported.
- Parameters:
Possible parms are :
Specifies how output cell values will be calculated when multiple rasters overlap.
kFME_Mosaic_overlappingValues_last
(Default) The last value.
kFME_Mosaic_overlappingValues_min
The minimum value.
kFME_Mosaic_overlappingValues_max
The maximum value.
kFME_Mosaic_overlappingValues_average
The average of all values.
kFME_Mosaic_overlappingValues_sum
The sum of all values.
kFME_Mosaic_overlappingValues_compositeUsingAlpha
Values will be blended based on the alpha band.
Yes or No (Default is No)
Specifies whether a nodata value can overwrite an existing real data value when they overlap. Only applies when mosaic_overlappingValues = last.
Yes or No (Default is No)
Specifies how palettes will be treated when present. When set to Yes, selected palettes will be merged to create a single palette for the output band. When set to No, selected palettes will be accumulated on the output band without modification.
- Return type:
- Returns:
The mosaicked raster.
- Raises:
FMEException – An exception is raised if an error occurred.
- offset(x, y, z, raster)
Performs offsetting on a raster.
If all the three x, y, z component values are 0 then no offsetting will be performed.
This method only works with numeric rasters without palettes.
- Parameters:
- Return type:
- Returns:
The raster after performing offsetting.
- Raises:
FMEException – An exception is raised if an error occurred.
- removeBandNodataValue(band)
Removes nodata value on the band.
- Parameters:
band (FMEBand) – Band object.
- Return type:
- Returns:
The band after removing nodata value.
- Raises:
FMEException – An exception is raised if an error occurred.
- replaceCellValues(minValue, maxValue, replacement, nodataFlag, band)
Replaces a range of value in the source raster with a new single value. All values greater than or equal to ‘minValue’ and less than or equal to ‘maxValue’ will be replaced by ‘replacement’.
Alternatively, if ‘minValue’ is greater than ‘maxValue’, then then the bounds define two distinct ranges that will be replaced by ‘replacement’. For example, a minValue of 200 and a ‘maxValue’ of 100 will replace all values that are greater than or equal to 200 or less than or equal to 100.
- Parameters:
minValue (FMETile) – Minimum value of band.
maxValue (FMETile) – Maximum value of band.
replacement (FMETile) – A new value that will replace existing one in the raster. That is, if
True
is specified, nodata values will be replaced just as any other values. IfFalse
is specified, then nodata values will not be replaced, even if they fall within the specified bounds.nodataFlag (bool) – Specifies whether nodata values will be replaced.
band (FMEBand) – Band object.
- Return type:
- Returns:
The band after replacing.
- Raises:
FMEException – An exception is raised if an error occurred.
- replaceCellValuesMultipleRanges(minValues, maxValues, replacements, nodataFlag, band)
Replaces ranges of values in the source raster with new values. In contrast to replaceCellValues(), this function can replace multiple ranges at once.
The ‘minValues’, ‘maxValues’, and ‘replacementValues’ must have the same number of entries.
When both minValue and maxValue have valid values, all source values >= minValue and <= to maxValue will be replaced with the corresponding replacement value. For example, if minValues[0] = 10, maxValues[0] = 20, and replacementValues[0] = 100, then all values >= 10 and <= 20 will be replaced with 100.
If minValue or maxValue is py:obj:None, then that range will be treated as one-sided. For example, if minValues[0] = 10, maxValues[0] is py:obj:None, and replacementValues[0] = 100, then all values >= 10 will be replaced with 100.
If a minValue is greater than its corresponding maxValue, this will be treated as two ranges. For example, if minValues[0] = 20, maxValues[0] = 10, and replacementValues[0] = 100, then all values >= 20 or <= 10 will be replaced with 100.
- Parameters:
minValues (list[int] or list[float] or list[None]) – Minimum values of band.
maxValues (list[int] or list[float] or list[None]) – Maximum values of band.
replacement – New values that will replace the existing ones in the raster that fall within the defined ranges.
nodataFlag (bool) – Specifies whether nodata values will be replaced. That is, if
True
is specified, nodata values will be replaced just as any other values. IfFalse
is specified, then nodata values will not be replaced, even if they fall within the specified bounds.band (FMEBand) – Band object.
- Return type:
- Returns:
The band after replacing.
- Raises:
FMEException – An exception is raised if an error occurred.
- reproject(sourceCSName, destCSName, interpolation, cellResize, raster, parms)
Performs coordinate system reprojection on a raster.
Specifying the same destination and source values will cause no processing to occur. Specifying a source coordinate system that is not the coordinate system of the source raster will cause the source raster to be treated as if it had the specified coordinate system; reprojection will occur, but the results may not be meaningful.
- Parameters:
sourceCSName (str) – FME coordinate system name that the raster is currently tagged with.
destCSName (str) – Desired coordinate system of the raster.
interpolation (int) –
Interpolation type of the raster. Choose one of:
FME_INTERPOLATION_NEARESTNEIGHBOR
FME_INTERPOLATION_BILINEAR
FME_INTERPOLATION_BICUBIC
FME_INTERPOLATION_AVERAGE4
FME_INTERPOLATION_AVERAGE16
FME_INTERPOLATION_SOURCE
cellResize (int) –
Specifies how the cell size of the output raster will be calculated. Choose one of:
FME_CELL_RESIZE_PRESERVE_NUM_CELLS
FME_CELL_RESIZE_SQUARE_CELLS
FME_CELL_RESIZE_PRESERVE_CELL_SHAPE
raster (FMERaster) – Raster to be processed.
parms (dict[str, str]) – (Optional) Specifies name-value pairs representing additional parameters. Currently, no additional parameters exist.
- Return type:
- Returns:
The raster after reprojection.
- Raises:
FMEException – An exception is raised if an error occurred.
- resampleByRowCol(numRows, numCols, interpolation, raster, parms)
Performs resampling (both upsampling and downsampling) on a raster using number of rows and columns.
The number of rows and number of columns dictate the desired size of the raster after processing. Valid input values are positive integers. The new X / Y spacing of the raster will be determined based on the input rows and columns and the extents of the raster in ground coordinates.
- Parameters:
numRows (int) – Desired number of rows of the raster after processing.
numCols (int) – Desired number of columns of the raster after processing.
interpolation (int) –
Interpolation of the raster. Choose one of:
FME_INTERPOLATION_NEARESTNEIGHBOR
FME_INTERPOLATION_BILINEAR
FME_INTERPOLATION_BICUBIC
FME_INTERPOLATION_AVERAGE4
FME_INTERPOLATION_AVERAGE16
FME_INTERPOLATION_SOURCE
raster (FMERaster) – Raster to be processed.
parms (dict[str, str]) – (Optional) Specifies name-value pairs representing additional parameters.
Possible parms are :
Valid arguments for this option are floating point values. If specified, the x origin of the resampled raster is adjusted by the provided value. The sample locations used when determining the data values of the output raster will also be offset by this value. This is useful when a raster needs to be accurately aligned to a reference grid. In order to more quickly adjust a raster origin without modifying data values, use the
offset
function.
This parameter is identical to resample_SnapOffsetX, except that it adjusts the y origin.
- Return type:
- Returns:
The raster after resampling.
- Raises:
FMEException – An exception is raised if an error occurred.
- resampleBySpacing(xSpacing, ySpacing, interpolation, raster, parms)
Performs resampling (both upsampling and downsampling) on a raster using ‘xSpacing’ and ‘ySpacing’.
The X / Y spacing in ground units dictates the desired size of the raster after processing. Valid input values are positive floating point numbers. The new number of rows columns of the raster will be determined based on the input spacing and the extents of the raster in ground coordinates.
- Parameters:
xSpacing (float) – Desired xSpacing of the raster after processing.
ySpacing (float) – Desired ySpacing of the raster after processing.
interpolation (int) –
Interpolation of the raster. Choose one of:
FME_INTERPOLATION_NEARESTNEIGHBOR
FME_INTERPOLATION_BILINEAR
FME_INTERPOLATION_BICUBIC
FME_INTERPOLATION_AVERAGE4
FME_INTERPOLATION_AVERAGE16
FME_INTERPOLATION_SOURCE
raster (FMERaster) – Raster to be processed.
parms (dict[str, str]) – (Optional) Specifies name-value pairs representing additional parameters.
Possible parms are :
Valid arguments for this option are floating point values. If specified, the x origin of the resampled raster is adjusted by the provided value. The sample locations used when determining the data values of the output raster will also be offset by this value. This is useful when a raster needs to be accurately aligned to a reference grid. In order to more quickly adjust a raster origin without modifying data values, use the
offset
function.
This parameter is identical to resample_SnapOffsetX, except that it adjusts the y origin.
- Return type:
- Returns:
The raster after resampling.
- Raises:
FMEException – An exception is raised if an error occurred.
- resolvePalettes(raster)
Resolves the values in a raster’s bands to the values in the lookup palettes associated with each band.
This method does not work with string palettes.
- Parameters:
raster (FMERaster) – The raster object where the bands belong to.
- Return type:
- Returns:
The raster after resolving the values.
- Raises:
FMEException – An exception is raised if an error occurred.
- rotate(rotationAngle, raster)
Adjusts the existing rotation angle on a raster by the specified ‘rotationAngle’.
The ‘rotationAngle’ specifies a counter clockwise (CCW) angle of rotation in degrees where 0 degrees is at 3 o-clock. Negative rotations are acceptable. Angles will be adjusted to be between 0 (inclusive) and 2PI (exclusive).
If the ‘rotationAngle’ is equal to 0 then no rotation will be performed.
- Parameters:
- Return type:
- Returns:
The raster after rotation.
- Raises:
FMEException – An exception is raised if an error occurred.
- scale(x, y, z, raster, parms)
Performs scaling on a raster.
If all the three ‘x’, ‘y’, and ‘z’ values are zero then no scaling will be performed.
- Parameters:
x (int) – Scaling factor for the x-spacing (and optionally origin) of the raster.
y (int) – Scaling factor for the y-spacing (and optionally origin) of the raster.
z (int) – Scaling factor for the data values of the raster.
raster (FMERaster) – Raster Object to apply scaling to.
parms (dict[str, str]) – (Optional) Specifies name-value pairs representing additional parameters.
kFME_RasterScale_scaleSpacingOnly
Yes or No (Default is No)
Specifies whether the scaling should affect only the spacing, or all raster properties (i.e. spacing, origin, rotation). Affecting all raster properties means scaling a raster will behave similarly to scaling all other geometries.
- Return type:
- Returns:
The raster after scaling.
- Raises:
FMEException – An exception is raised if an error occurred.
- setBandNodataValue(nodataValue, replaceFlag, band)
Sets the nodata value on the band.
- Parameters:
nodataValue (FMETile) – New nodata value.
replaceFlag (bool) – Specifies whether the old value should be replaced with new value. When it is
False
, the band will be tagged with the new ‘nodataValue’. When it isTrue
, not only will the band be tagged with the new ‘nodataValue’, but also any cells with the previous nodata value will be replaced by the new ‘nodataValue’.band (FMEBand) – Band object.
- Return type:
- Returns:
The band after setting the nodata value on the band.
- Raises:
FMEException – An exception is raised if an error occurred.
- subset(startRow, numRows, startCol, numCols, paddingTop, paddingLeft, paddingBottom, paddingRight, raster, parms)
Performs clipping of a raster using pixel bounds rather than ground coordinates.
- Parameters:
startRow (int) – Starting row. Value cannot be negative number.
numRows (int) – Total number of rows of the new raster. Value must be greater than 0.
startCol (int) – Starting column. Value cannot be negative number.
numCols (int) – Total number of columns of the new raster. Value must be greater than 0.
paddingTop (int) – Number of rows of padding on the top of the subset portion of the raster.
paddingBottom (int) – Number of rows of padding on the bottom of the subset portion of the raster. Value cannot be negative number.
paddingRight (int) – Number of columns of padding on the right of the subset portion of the raster. Value cannot be negative number.
paddingLeft (int) – Number of columns of padding on the left of the subset portion of the raster. Value cannot be negative number.
raster (FMERaster) – The raster Object to apply clipping to.
parms (dict[str, str]) – (Optional) Specifies name-value pairs representing additional parameters. Currently, no additional parameters exist.
- Return type:
- Returns:
The raster after clipping.
- Raises:
FMEException – An exception is raised if an error occurred.