fmeobjects.FMEUniversalReader
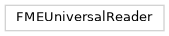
Terminate the reader mid-stream. |
|
Close the reader after it has exhausted its data source. |
|
This method flushes cached geometries to disk. |
|
Query the native reader for properties. |
|
Return property values for the given property category. |
|
|
Open the reader. |
Return the next feature in the data source. |
|
Return the next schema feature for the data source. |
|
This method provides FME with a callback function that will be called while caching features from a dataset. |
|
|
Specify the spatial and attribute constraints to be used when reading the data. |
|
Extract reader parameters from schema feature and set the parameters on this reader. |
- class FMEUniversalReader
FME Universal Reader Class
init(readerName, cacheFeatures, directives)
Create a FME reader to read data from a format or system. Only the reader’s format identifier, as found in the format index need be passed in. Any parameters needed for the reader will be passed to it via its ‘open’ call.
- Parameters:
readerName (str) – Reader’s format identifier
cacheFeatures (bool) – If
True
then the reader builds a spatial index and caches the features enabling subsequent reads to be much faster. Only set toTrue
when the input data is to be read multiple times. Set toFalse
if each feature from the source dataset is only read once.directives – Specify one or more additional directives to the reader. All elements in this collection must be
str
. A list should be used if one key is to be set to multiple values. If a list is passed in, key-value pairs such as those in a dict must be placed side by side. For example, the key should be placed in the first list element, followed by the value for the key. The supported directives are:
USER_PIPELINE
: This directive takes a pipeline file name as its values. If it is not a full path name then the pipeline file must be in the userPipeline directory under the FME installation. If it is an absolute path name then the pipeline file at that location is opened and used. Specifying this directive results in all features from the reader first going through the user pipeline before being given to the application.RUNTIME_MACROS
: This directive takes a System Data Format (SDF) list of macro names and values – see fmedlg.h for a description. This directive provides a convenient means for settings to be propagated from the dialog box package to the reader being created. These settings control how the reader itself will behave.META_MACROS
: This directive takes a System Data Format (SDF) list of macro names and values – see fmedlg.h for a description. This directive provides a convenient means for settings to be propagated from the dialog box package to the reader being created. These settings typically govern how the reader’s metafile and schema information will behave.METAFILE
: This directive takes the name of the metafile that should be used for this reader. This is returned in the metafile parameter from the dialog box package. Occasionally the settings box may request a specific metafile be used to control the reader’s behaviour. This directive provides a means for propagating this information to the reader.COORDSYS
: This directive takes the name of the coordinate system that all features should be converted to (if the reader thinks they are from a different coordinate system), or tagged with. The value is any valid FME coordinate system name. This allows the caller to dictate the coordinate system that the features should be returned in.IDLIST
: This directive takes a SDF formatted list of ‘IDs’ which will limit the tables being read by the reader. This allows the caller to limit the tables that will be read in. For example, the list may contain the basenames of the shape files which should be read from a directory.DEFLINE
: This directive takes an unparsed character string which contains an entire DEF line to add to any others which will be used. (The value should not include the first ‘KEYWORD_DEF’ token.) This allows the caller to specify DEF lines that the reader should use in conjunction with any others that may be automatically read from the ‘metafiles/KEYWORD_reader_def.fmi’ file.OUTPUT_STATS
: This directive takes a value of YES or NO. If NO, then no writer statistics are output to the log file. The default is YES. This allows the caller to turn off some statistic logging that writers normally do.SCHEMA_FORMAT_ATTRIBUTES
: This directive takes a value of YES or NO. If YES, then any readSchema calls on the reader will contain the format attributes for that reader on the returned schema features. The default is NO.--macro_name
: Any directive that begins with two dashes is considered to be a macro name that will be set for use within the canned DEF files used by the reader (<readerName>_reader_def.fmi). For example, if a directive name ‘–MySetting’ with a value of ‘Yes’ is given, then the macro ‘MySetting’ will be given a value of ‘Yes’, for use within the DEF file.
- __init__(*args, **kwargs)
- abort()
Terminate the reader mid-stream.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- close()
Close the reader after it has exhausted its data source.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- flushCachedGeometries()
This method flushes cached geometries to disk. When reading and building a feature cache, most geometries will be kept on memory to avoid the expense of serializing them. Calling this method forces the unserialized geometries to be written to disk. Typically it is not necessary to make this call. However, it may be useful in cases where the temporary cached ffs file is being accessed directly.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- getNativeProperties(propertyCategory)
Query the native reader for properties. It is a proxy to the pluginbuilder.FMEUniversalReader.getProperties() method. For more information, please consult the documentation for pluginbuilder.FMEUniversalReader.getProperties() in the FME Plugin Builder SDK.
- getProperties(propertyCategory)
Return property values for the given property category.
This method handles the following property categories:
'fme_prop_spatial_index'
: The return value will contain the key ‘fme_prop_spatial_index’ with eitherTrue
or ‘False
’ indicating if the reader being used is spatially enabled or not.None
is returned if the reader does not know the correct value.'fme_prop_persistent_cache_loaded'
: The return value will contain the key ‘fme_prop_persistent_cache_loaded’ with eitherTrue
or ‘False
’ indicating if the reader persistent cache is loaded or not.None
is returned if the reader does not know the correct value.'fme_prop_coord_sys_aware'
: The return value will contain the key ‘fme_prop_coord_sys_aware’ with eitherTrue
or ‘False
’ indicating if the reader is coordinate system aware or not.None
is returned if the reader does not know the correct value.'*'
: Returns all properties for all known property categories.
Refer to IFMEReader setConstraints() Documentation for more additional information.
- open(datasetName, parameters)
Open the reader.
- Parameters:
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- read()
Return the next feature in the data source.
- Return type:
- Returns:
Returns
None
if the data source is exhausted.- Raises:
FMEException – An FMEException is raised if there was an error.
- readSchema()
Return the next schema feature for the data source.
- Return type:
- Returns:
Returns
None
if all the schema features have been returned.- Raises:
FMEException – An FMEException is raised if there was an error.
- setCachingCallback(function, frequency)
This method provides FME with a callback function that will be called while caching features from a dataset.
Caching of an uncached dataset will occur when
setConstraints()
is used for reading a dataset or when a reader is set to cache on schema read.In the
setConstraints()
case, caching will start when the first feature is read.In the schema read case, caching will start when the first schema feature is read. A reader is set to cache on schema read by passing in the
kFME_ReadCachedSchema
key/value pair in the creation directives for theFMEUniversalReader
.The callback method should return a
bool
, whereTrue
means to continue caching andFalse
means to stop. Stopping does not invalidate the cache. It will still be functional, but only up to the number of features cached. The method should also accept anint
parameter that will specify the number of features that have been cached up to that point. e.g. callbackFunction(numFeaturesCached)The rate FME calls the callback function will be determined by the frequency parameter, which is the number of features cached per call to the callback. In some cases, FME may cache more than the frequency parameter in a single cache operation. In those cases, the callback will be called as close to the frequency as possible.
Specifying a callback function of
None
will turn off the callback.Exceptions raised by the callback method will mean the equivalent of a
False
return value.- Parameters:
function (Callable) – The function to provide FME for use as a callback function.
frequency (int) – The frequency of calling the callback method based on the number of features cached.
- Return type:
None
- setConstraints(feature)
Specify the spatial and attribute constraints to be used when reading the data. The spatial and attribute query is specified in the feature object. This only need be implemented by those readers which return spatialEnables() as true, otherwise they can do nothing. This can be called at any time after the reader is created. If any read is in progress then it is terminated and the next read will reflect the new constraints.
The constraint feature must have an attribute called ‘fme_search_type’ on it. The value of this attribute indicates the kind of search to be done. Refer to the IFMEReader setContraints() Documentation for detailed information.
- Parameters:
feature (FMEFeature) – The constraint feature.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.
- setSchema(feature)
Extract reader parameters from schema feature and set the parameters on this reader.
- Return type:
None
- Raises:
FMEException – An FMEException is raised if there was an error.