fmeobjects.FMERegex
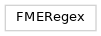
|
Return all non-overlapping matches in string, as a list of str. |
|
Return a list yielding match objects over all non-overlapping matches in string. |
|
If zero or more characters at the beginning of string match this regular expression, return a corresponding match tuple. |
|
Scan through string looking for the first location where this regular expression produces a match, and return a corresponding match object. |
- class FMERegex
FME Regex Class
init(regexPattern)
This class provides FME regular expression functionality that is differs from Python’s built-in re module.
- Parameters:
regexPattern (str) – Regular expression pattern.
- Return type:
- Returns:
An FMERegex object.
- __init__(*args, **kwargs)
- findall(inputString)
Return all non-overlapping matches in string, as a list of str. The string is scanned left-to-right, and matches are returned in the order found.
- findlist(inputString)
Return a list yielding match objects over all non-overlapping matches in string. The string is scanned left-to-right, and matches are returned in the order found.
- Parameters:
inputString (str) – Input string to match.
- Return type:
- Returns:
A list of match tuples of the form (match, start, end), where match is the string segment matching the regular expression pattern, start is the start index of the string segment, and end is the end index of the string segment.
- match(inputString)
If zero or more characters at the beginning of string match this regular expression, return a corresponding match tuple.
- Parameters:
inputString (str) – Input string to match.
- Return type:
- Returns:
A match tuple of the form (match, start, end), where match is the string segment matching the regular expression pattern, start is the start index of the string segment, and end is the end index of the string segment. None is returned if a match is not found.
- search(inputString)
Scan through string looking for the first location where this regular expression produces a match, and return a corresponding match object.
- Parameters:
inputString (str) – Input string to match.
- Return type:
- Returns:
A match tuple of the form (match, start, end), where match is the string segment matching the regular expression pattern, start is the start index of the string segment, and end is the end index of the string segment. None is returned if a match is not found.