fmeobjects.FMELicenseManager
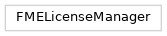
Retrieves the value of a property from a 3rd party license file. |
|
Returns all the factory names for the current license. |
|
Returns all the format names for the current license. |
|
Returns all the function names for the current license. |
|
Retrieves the value of a property for a given application. |
|
Retrieves the value of a property for a given factory. |
|
getFactoryMinBaseCapability(factoryName), |
|
Retrieves the value of a property for a given function. |
|
Returns the minimum base capability for the specified function to be licensed. |
|
|
Retrieves the value of a property from the current license. |
Returns the current license type. |
|
This routine returns the maximum number of licensed fme processes. |
|
This routine returns the maximum number of licensed fmeworker processes. |
|
Retrieves the value of a property for a given reader. |
|
Returns the minimum base capability for the specified reader to be licensed. |
|
Retrieves the value of a property for a given writer. |
|
Returns the minimum base capability for the writer factory to be licensed. |
|
|
Returns whether or not the minimum base capability is met. |
|
Returns whether or not an application is licensed. |
|
Returns whether or not a given ArcGIS product is installed. |
|
Returns whether or not a given factory is licensed. |
Returns whether or not a given function is licensed. |
|
Returns whether or not the Gtrans reprojection library is installed. |
|
|
Returns whether or not a given reader is licensed. |
|
Returns whether or not a given writer is licensed. |
This method is used to validate a license file. |
- class FMELicenseManager
FME License Manager Class
init()
This class provides an interface to security functionality.
- __init__(*args, **kwargs)
- get3rdLicenseProperty(licenseFileName, vendorID)
Retrieves the value of a property from a 3rd party license file.
- Parameters:
licenseFileName (str) – The file name of a 3rd party license file.
vendorID (int) – The custom key assigned by the vendor, used to verify that the property is licensed.
vendorRegKey (int) – The registration code of the vendor assigned by Safe Software, used to verify that the property is licensed.
property (str) – The name of the property to be retrieved.
- Return type:
str or None
- Returns:
The value of the property if successful or
None
otherwise.
- getAllFactories()
Returns all the factory names for the current license. For all factories the list will be populated with <factory name>/<license value> pairs. <license value> can be either
kFME_FormatLicensed
orkFME_FormatUnlicensed
.
- getAllFormats()
Returns all the format names for the current license. For all formats the list will be populated with <format name>/<license value> pairs. <license value> can be one of the following:
kFME_FormatRead
: Only reader is licensed.kFME_FormatWrite
: Only writer is licensed.kFME_FormatBoth
: Both reader and writer are licensed.kFME_FormatUnlicensed
: Neither reader nor writer is licensed.
- getAllFunctions()
Returns all the function names for the current license. For all functions the list will be populated with <function name>/<license value> pairs. <license value> can be either
kFME_FormatLicensed
orkFME_FormatUnlicensed
.
- getAppLicenseProperty(appName, property)
Retrieves the value of a property for a given application. This is only used for application in which there are different levels of support.
- getFactoryLicenseProperty(factoryName, property)
Retrieves the value of a property for a given factory. This is only used for factories in which there are different levels of support.
- getFactoryMinBaseCapability()
getFactoryMinBaseCapability(factoryName),
Returns the minimum base capability for the specified factory to be licensed. Will return one of the following capability names:
DESKTOP
PROFESSIONAL
ENTERPRISE_GIS
ENTERPRISE_DB
SERVER
SMALLWORLD
FORM
NATIONAL
BASIC_RASTER
ADVANCED_RASTER
EXTRA_COST
- getFunctionLicenseProperty(functionName, property)
Retrieves the value of a property for a given function. This is only used for function in which there are different levels of support.
- getFunctionMinBaseCapability(functionName)
Returns the minimum base capability for the specified function to be licensed. Will return one of the following capability names:
DESKTOP
PROFESSIONAL
ENTERPRISE_GIS
ENTERPRISE_DB
SERVER
SMALLWORLD
FORM
NATIONAL
BASIC_RASTER
ADVANCED_RASTER
EXTRA_COST
- getLicenseProperty(property)
Retrieves the value of a property from the current license.
- getLicenseType()
Returns the current license type. Possible values are:
MACHINE SPECIFIC
OEM
GENERIC
FLOATING.
- getMaxFMEProcesses()
This routine returns the maximum number of licensed fme processes.
- Return type:
- Returns:
Returns the maximum number of licensed fme processes.
- getMaxFMEWorkerProcesses()
This routine returns the maximum number of licensed fmeworker processes.
- Return type:
- Returns:
Returns the maximum number of licensed fmeworker processes.
- getReaderLicenseProperty(readerName, property)
Retrieves the value of a property for a given reader. This is only used for reader in which there are different levels of support.
- getReaderMinBaseCapability(readerName)
Returns the minimum base capability for the specified reader to be licensed. Will return one of the following capability names:
DESKTOP
PROFESSIONAL
ENTERPRISE_GIS
ENTERPRISE_DB
SERVER
SMALLWORLD
FORM
NATIONAL
BASIC_RASTER
ADVANCED_RASTER
EXTRA_COST
- getWriterLicenseProperty(writerName, property)
Retrieves the value of a property for a given writer. This is only used for writer in which there are different levels of support.
- getWriterMinBaseCapability(writerName)
Returns the minimum base capability for the writer factory to be licensed. Will return one of the following capability names:
DESKTOP
PROFESSIONAL
ENTERPRISE_GIS
ENTERPRISE_DB
SERVER
SMALLWORLD
FORM
NATIONAL
BASIC_RASTER
ADVANCED_RASTER
EXTRA_COST
- hasBaseCapability(capability)
Returns whether or not the minimum base capability is met.
- isAppLicensed(appName)
Returns whether or not an application is licensed.
- isArcGISInstalled(productName)
Returns whether or not a given ArcGIS product is installed. The following products are supported:
ArcGIS Desktop
ArcGIS Engine
ArcGIS Server
None
- any ArcGIS product
- isFactoryLicensed(factoryName)
Returns whether or not a given factory is licensed.
- isFunctionLicensed(functionName)
Returns whether or not a given function is licensed.
- isGtransInstalled()
Returns whether or not the Gtrans reprojection library is installed.
- isReaderLicensed(readerName)
Returns whether or not a given reader is licensed.
- isWriterLicensed(writerName)
Returns whether or not a given writer is licensed.
- validateLicenseFile(licenseFilePath)
This method is used to validate a license file. Note: It is not using the license file but rather merely returning whether or not the license file is valid for the current machine if it is installed.