fmeobjects.FMEReprojector
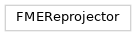
Get the coordinate systems being used. |
|
Retrieve the last error message if an exception had been raised internal to this class. |
|
Returns |
|
|
This reprojects the angle of the given line, measured CCW from the horizontal, and returns the resulting angle in degrees. |
|
This reprojects the given list of coordinates, and returns the results. |
|
This reprojects the given feature using the coordinate systems currently set. |
|
This reprojects the given geometry using the coordinate systems currently set. |
|
This reprojects the 2D length of the given line, returning the length in ground units. |
Set the coordinate systems being used. |
|
Check if the currently set coordinate systems are valid. |
- class FMEReprojector
FME Reprojector Class
Creates a new reprojector object.
init()
Constructs a reprojector object. Not specifying any directives results in FME choosing a default engine based on the context, as well as what engines are licensed. If none are licensed, an exception will be thrown.
- Return type:
- Returns:
This method returns a new reprojector object.
init(directives)
Constructs a reprojector object.
- Parameters:
directives (list[str]) – Additional directives to specify for the reader. Directives are of the form of a directive name as an entry in the list, with the next entry having the value for the directive. An empty list of str can be used to specify that no directives are present. The supported directives are : REPROJECT_ENGINE This directive takes a reprojection engine name as its values. If it is not a valid name, or specifies an engine that is not licensed, an exception will be thrown.
- Return type:
- Returns:
This method returns a new reprojector object.
- __init__(*args, **kwargs)
- getCoordinateSystems()
Get the coordinate systems being used. If the coordinate systems are invalid or were not set, empty strings will be returned.
- getLastErrorMessage()
Retrieve the last error message if an exception had been raised internal to this class. A blank str is returned if there was no previous error message.
- Return type:
- Returns:
The last error message.
- nullReprojection()
Returns
True
if the reprojection will not alter coordinate values andFalse
otherwise. For example, this might occur if either the source or destination coordinate system is non-earth.- Return type:
- Returns:
Whether or not the reprojection will alter coordinate values.
- reprojectAngle(startX, startY, finishX, finishY)
This reprojects the angle of the given line, measured CCW from the horizontal, and returns the resulting angle in degrees. If the coordinate systems are not set or invalid, this will throw an exception, and the result will be set to the original angle.
- Parameters:
- Return type:
- Returns:
The angle of the reprojection in degrees.
- Raises:
FMEException – An exception is raised if an error occurred.
- reprojectArray(xyzCoords)
This reprojects the given list of coordinates, and returns the results. This will throw an exception if the coordinate systems are not set or invalid, or if the reprojection cannot be completed.
- Parameters:
xyzCoords (list[tuple[float]]) – The list of coordinates. xyzCoords is a list of tuples in the form of [(x[0], y[0], z[0]), (x[1], … , (x[n-1], y[n-1], z[n-1])] with n being the number of xyz coordinate tuples.
- Return type:
- Returns:
The given result of the reprojection.
- Raises:
FMEException – An exception is raised if an error occurred.
- reprojectFeature(feature)
This reprojects the given feature using the coordinate systems currently set. This honours any pre-reproject functions set. If the feature has a source coordinate system set, it will be used instead of the source coordinate system set on the current reprojector. If
None
is passed in,None
is returned.- Parameters:
feature (FMEFeature or None) – The feature to reproject.
- Return type:
FMEFeature or None
- Returns:
The input feature after reprojection.
- Raises:
FMEException – An exception is raised if an error occurred.
- reprojectGeometry(geometry)
This reprojects the given geometry using the coordinate systems currently set. The type of geometry returned may not be the same as the geometry given. For instance, if a
FMEArc
was given, aFMELine
may be returned. This will throw an exception if the coordinate systems are not set or invalid, or if the reprojection cannot be completed. IfNone
is passed in,None
is returned.- Parameters:
geometry (FMEGeometry or None) – The geometry to reproject.
- Return type:
Same type as input geometry.
- Returns:
The input geometry after reprojection.
- Raises:
FMEException – An exception is raised if an error occurred.
- reprojectLength(startX, startY, finishX, finishY)
This reprojects the 2D length of the given line, returning the length in ground units. If the coordinate systems are not set or invalid, this will return an error, and results will be set to the original length.
- Parameters:
- Return type:
- Returns:
The length of the reprojection in ground units.
- Raises:
FMEException – An exception is raised if an error occurred.
- setCoordinateSystems(sourceCS, destCS)
Set the coordinate systems being used. Will throw an exception if the coordinate systems cannot be set or are invalid.
- Parameters:
- Return type:
None
- Raises:
FMEException – An exception is raised if an error occurred.